Start automating your tests 10X Faster in Simple English with Testsigma
Try for freeAPI (Application Programming Interface) is the building block of software applications. More often than not, developers use APIs to access data, facilitate interaction between multiple applications, enable communication between Microservices, etc. So, in this blog post, we will cover the API testing basics. Let’s begin!
Table Of Contents
- 1 What is API Testing?
- 2 API Facets
- 3 How are API called?
- 4 API Request Method
- 5 API Response
- 6 API Gateway
- 7 API Authentication
- 8 Generating Test cases for API testing
- 9 API Testing Approach
- 10 How to Test API?
- 11 Best Practices of API Testing
- 12 Challenges of API Testing
- 13 Conclusion
- 14 Frequently Asked Questions
- 14.1 How will I get to know which API follows what method?
- 14.2 Can one API endpoint perform multiple actions?
- 14.3 Can I use API without specifying the method?
- 14.4 Can all APIs be accessed through a URL?
- 14.5 Can the same endpoint have multiple methods associated?
- 14.6 What are the three layers of API testing?
- 14.7 What is the process of API testing?
- 14.8 What is Postman API?
- 15 Suggested Readings
What is API Testing?
API testing is integral to software development to provide optimal application performance. API, a specialized application programming interface, is a collection of protocols, tools, and functions that facilitate seamless communication between software applications.
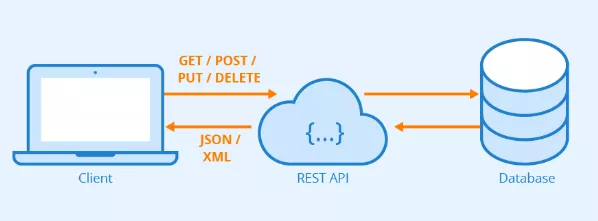
While many software testers perceive working with API as a frightening experience, APIs are the simplest and most non-complicated way of dealing with applications. This article unveils the basic concepts of API testing, intending to make one feel confident and comfortable interacting with APIs.Let us take the first step towards understanding Web APIs with the bird’s view of the client-server diagram. Web application designers use this infamous client-server setup to create a comprehensive picture.
Client/ client-side- includes all the devices used to interact with the application.
The Internet is an enabler; it assists in transporting client requests to the Web server along with relevant details filled in by the user on the client side.
Web-server/server-side is the holy place where all processing takes place. Once the processing is complete, the server pushes the data back to the client.
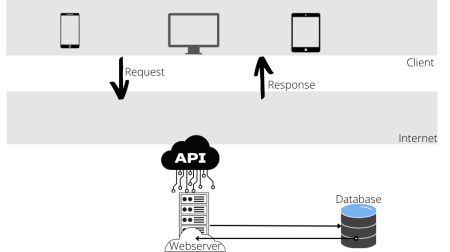
API Facets
APIs comes with 2 broad facets
1. Request– An encapsulation of action performed along with pertinent details to be sent to the server.
2. Response– An encapsulation of processed data along with supplement details sent back to client by the server.
Both request and response come with header attached. Take a look at any network call, you should be able to see headers quite evidently.
The Request header passes all the additional details to the server to process the Request. In the example below, we see a set of keys and values. The key names are case insensitive.
Sometimes Request also carries payload details; in the below example, I am trying to join the Testsigma discord community. To make that happen, I entered some details through UI and clicked on the “Send” button. In the backend call, we can see that the details passed to the server when the event is triggered fill the payload.
A web server processes the Request and prepares the response body and additional header details. For example, when I chose to join the discord community, the API responded with further information about filling captcha. We plan to perform regressive testing on the response body during API testing to verify that the sent details match our expectations.
How are API called?
Each user interaction on UI is associated with an API that will be accessed through a URL. Let us say a user fills up a form and clicks on “Send” button, on trigger of this event respective API will be invoked. In the below picture we can see the Request URL is pointing to the endpoint ‘Signup’.
API Request Method
Every request is associated with the “Request Method”. This method specifies the desired action to be performed by the server. Below are the frequently used methods
1. GET: This method is used to retrieve the details, this performs a read-only operation.
2. POST: This method submits data by creating an entry into database. This method should be used wisely as it can change the state causing side effects if not handled properly.
3. PUT: This method is used to update any data, the data that needs to be replaced is sent as part of payload.
4. DELETE: This method just deletes the data.
5. HEAD: This is similar to get method, however there is no response body sent.
6. PATCH: This method specifies how to update the data, this can can cause side effects if not handled with care.
Few FAQs about request methods
1. How will I get to know which API follows what method?
Ideally software development teams maintain API documentations. Every API is preceded with the respective method in the documentation.
2. Can one API endpoint perform multiple actions?
Yes, sometimes same endpoint is designed to do multiple methods.
3. Can I use API without specifying method?
No, you have to suffice the API call with request method
4. Can all APIs be accessed through URL
Yes and NO, some APIs are open for public and may not need authentication. However most of the business and enterprise APIs are behind API gateway and one may have to authenticate before calling the API.
5. Can same endpoint have multiple method associated?
Yes, it is completely possible to define multiple actions/verbs for same endpoint. Hence it is important to know before hand what actions are supported by the API.
API Response
Every API request is associated with response. The response have 2 facets
1. Status code : Numeric representation of the web server response. There are pre-defined set of status code which can be reused. Or, teams can create their own status code as per convenience. However, appropriate status code range should be used.
2. Status Message : Each status code is associated with detailed response message.
for example:
Status code 200 → Success
Status code 401 → Unauthorized
Status code 404 → Not found
API Gateway
API usage is not as simple in real world, when companies are hosting large scale APIs protecting them from misuse is their primary concern. API gateway helps establishing authentication to verify the calls before transferring it to further execution. The capability of API gateway is not just restricted to authentication, it also provides multitude of services like
I. Routing
ii. Rate Limiting
iii. Analytics
iv. Security
v. Policies etc
API Authentication
Since APIs deal with protected resources, the request processing should be backed with authentication process to ensure the access is provided only to the intended user. There is a difference between authentication and authorisation.
Authentication is a process of verifying the identity of the user it solely answers who you are, whereas authorisation mostly deals with access management and comes into play only after the user is identified and verified successfully.
Most commonly used authentication are
1. Basic Authentication:
This technique involve providing username and password for user verification. Authentication sounds little flimsy isn’t it?, but this is how it is going to work. When user enters their credentials the details are encoded in Base64 generating a Key which will be bundled in request header and sent to server for verification. Server Verifies the key with the stored
username and password. If the identity is verified the request is fulfilled else an error is sent back denying request sent.
2. API Key Authentication :
API key is a long encrypted string which identifies the application without any principal. These are sent either as a part of request header or URL. When client recognises the API key server will process the request.
3. OAUTH Authentication :
This technique is considered quite powerful and secure way of authenticating the users. oAuth technique can also be used for authorisation. Initially a user may have to login to the oAuth application using the credentials to generate a token. The generated token is attached as part of request header, which will be sent to authentication server in order to verify. If the token is recognised the API request will be processed
Generating Test cases for API testing
API testing is a black box testing technique, one don’t really delve into what is implemented within an API. Having said that, if you would like to particularly walk through the code to test the API that is fine too. The main task in testing APIs is to figure out the test cases just like any other tests out there.
For example consider the below REST API
GET https://gorest.co.in/public/v2/users
Looking at the API method we understand that this is going to perform a read operation and fetch the details requested which is list of all users. The response returned will be in the JSON format. REST Apis generally use JSON structure to represent the response body.
Let us go ahead churning the test cases for this API
1. On Successful execution the API should return status code 200 (message : Success)
2. Specific user details can be fetched by passing user id in URL (https://gorest.co.in/public/v2/users/25)
3. The JSON response should match the below schema
{
“id”:integer,
“name”:string,
“email”:string,
“gender”:string,
“status”:string
}
4. Wrong URL should respond with 404 (Not found)
5. Multiple User ID in the URL should respond with 404 error
API Testing Approach
There are various approaches to API testing, and the following points highlight some of the standard testing approaches:
- Functional testing: This involves testing the functionality of the API to ensure that it meets the specified requirements.
- Security testing: This involves testing the security of the API to ensure that it is not vulnerable to security threats.
- Load testing: This involves testing the performance of the API under different loads to ensure that it can handle the expected traffic.
- Integration testing: This involves testing the integration of the API with other software components to ensure that it works seamlessly.
Overall, a comprehensive API testing approach should cover all these testing approaches to ensure the quality and reliability of the software application.
How to Test API?
- Understand the API’s Functionality
Before you start testing an API, you need to understand its functionality. This includes the data it exchanges with the client, the expected format of the data, the input parameters, and the expected output. Understanding the API’s functionality will help you design your test strategy and ensure that your test cases cover all the required scenarios.
- Test the API Endpoints
Once you understand the API well, you can begin testing the endpoints. This involves sending requests to the API using various HTTP methods (GET, POST, PUT, DELETE).
Best Practices of API Testing
The best practices of API testing are essential to ensure the functionality and reliability of an application programming interface.
- Plan and design the API tests before starting the testing process.
- Use a testing framework that provides robust features to automate API testing.
- Create test data covering a wide range of scenarios and edge cases.
- Verify the API’s functional behavior, security, and performance.
- Conduct regression testing to ensure that changes and updates do not impact the existing API functionality.
- Integrate API testing with continuous integration and delivery pipelines.
- Collaborate with developers and other stakeholders to identify and resolve issues early.
- Use monitoring tools to track API performance and identify issues before they impact end-users.
Challenges of API Testing
Here are some of the most common challenges:
- Complex API architecture and integration with other systems can make testing difficult and time-consuming.
- API testing requires programming skills and knowledge of HTTP protocols and methods.
- Test data management can be challenging, especially when dealing with large data sets.
- Ensuring API security and authentication is essential, but testing can be challenging.
- API testing requires close collaboration between developers, testers, and stakeholders to ensure that the APIs meet the business requirements and user expectations.
Conclusion
Hey, it’s time to wrap things up! API testing is super important when it comes to software development. It ensures that APIs play nice with other systems and do what they should. If you’re new to the game, getting a handle on the API testing basics, including the different tests, tools, and tricks involved, is key. This guide should give you a solid overview of API testing basics, so you can confidently start testing your APIs. But listen up: API testing isn’t a one-and-done deal; it’s an ongoing process that you must keep up with to keep your APIs in tip-top shape. Keep learning, keep testing, and keep improving those APIs!
Happy Testing !
Frequently Asked Questions
How will I get to know which API follows what method?
The request header carries all the supplemental details to be passed on to the server to process the Request. In the example below, we see a set of keys and values. The key names are case insensitive.
Can one API endpoint perform multiple actions?
Yes, sometimes the same endpoint is designed to do multiple methods.
Can I use API without specifying the method?
No, you have to suffice the API call with the request method
Can all APIs be accessed through a URL?
Yes, and NO, some APIs are open to the public and may not need authentication. However, most business and enterprise APIs are behind API gateway, and one may have to authenticate before calling the API.
Can the same endpoint have multiple methods associated?
It is possible to define multiple actions/verbs for the same endpoint. Hence, it is essential to know beforehand what actions the API supports.
What are the three layers of API testing?
People commonly refer to the three layers of API testing as unit testing, integration testing, and end-to-end testing.
What is the process of API testing?
API testing typically involves identifying the requirements for the API, designing test cases, executing the tests, and analyzing the results.
What is Postman API?
Postman API is a platform that promotes the development, testing, and documentation of APIs.