In this blog post, we’ll delve into the details of this unit testing, including how it works, the benefits it offers, and tips for getting the most out of the process. Whether you’re new to this testing or looking to deepen your understanding of this important topic, this post has something for you. So let’s get started!
Table Of Contents
- 1 What is Unit Testing?
- 2 Why Unit Testing is Important?
- 3 What is the Objective of Unit Testing?
- 4 Unit Testing Examples
- 5 Who Should Create The Unit Test?
- 6 Unit Testing Techniques
- 7 How Unit Tests Work?
- 8 Types of Unit Testing
- 9 Unit Testing Techniques
- 10 Advantages and Disadvantages
- 11 Manual vs. Automated Unit Testing
- 12 How to Write Unit Test Cases
- 13 Workflow of Unit Testing
- 14 Unit Testing Myth
- 15 Unit Testing Best Practices
- 16 What are the Unit Testing Tools?
- 17 Conclusion
- 18 Frequently Asked Questions:
What is Unit Testing?
Unit testing is functional testing that verifies the individual units of source code. A team is the smallest testable part of an application. Being a part of the tech team at KingEssays, I should say that this testing is essential because it helps you verify that your code works as intended. It also allows you to find and fix problems with your code. You create a safety net for your code when you write unit tests. If something breaks, the unit tests will alert you so that you can fix the problem.
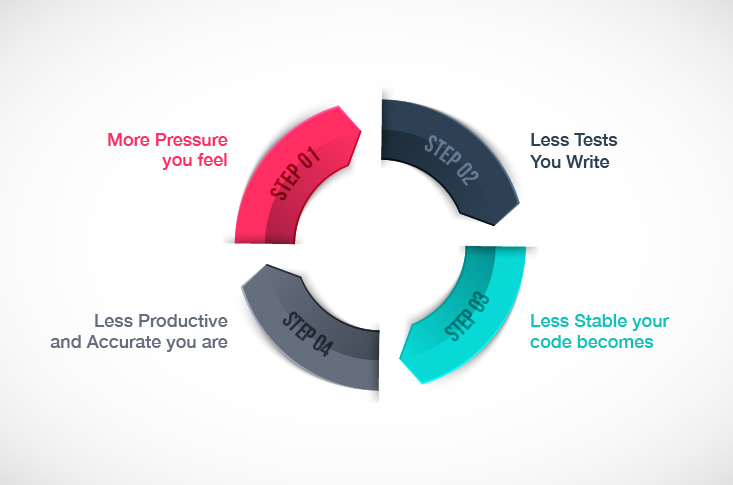
This testing is done either manually or with automated tools. Automated testing tools are often more reliable and efficient, but they can be expensive to set up and maintain.
Why Unit Testing is Important?
Unit testing is crucial to ensure software quality. It helps make sure that the software application meets its functional requirements and works as intended. Unit tests have a number of benefits,
- It helps improve the code quality
- As unit tests are done in the early stages of the development lifecycle, it helps detect and fix bugs soon.
- It enables code reusability
- It accelerates deployment velocity
What is the Objective of Unit Testing?
Unit testing is a vital software development exercise designed to ensure that each program component works correctly. The main objective of this testing is to ensure that any changes or updates to the code do not cause unexpected errors by verifying that all separate modules – like functions and procedures – are functioning correctly. Further, this testing aims to,
- Verify the code correctness
- Tests every function and module of an application
- Identify bugs in the early stages of the development cycle, which helps save costs.
- This testing helps developers understand their code base, which allows them to fix bugs quickly.
- Enables code reusability
- Since it helps detect coding errors very early, it helps save time and resources in the long run.
Unit Testing Examples
Let me explain a simple unit testing example: a function that adds two integers and returns the sum as the output.
Function
A unit test code will look like,
If the function returns anything other than 30, then it means the test case fails, and there is some issue with the code.
You can do this manually by just checking the sum manually. Instead, you can automate this process using unit testing tools like JUnit.
Who Should Create the Unit Test?
Developers who developed the code should create unit tests. That is because the developers would know better about the code’s functionality.
But, when unit tests are automated, both testers and developers will be involved while executing the unit tests.
Robert V. Head, in his book Real-time Business Systems, quoted, “Frequently, unit testing is considered part of the programming phase, with the person that wrote the program…unit testing”.
It makes so much sense. Who else other than the creators can understand the code? Proper unit testing helps improve the code’s accuracy and ensure it is bug-free.
Unit Testing Techniques
The three types of this testing techniques include,
Black Box Testing
Here, testers evaluate the functionality of units of code without knowledge of its internal implementation. Test cases are designed based on input-output specifications and help validate the external behavior.
White Box Testing
It helps examine the internal logic and structure of the code. Here, developers test the functional behavior of an application by providing input and reviewing the output.
Gray Box Testing
It is a combination of both white box and black box testing. Testers have partial knowledge of the internal workings of the code.
How Unit Tests Work?
Unit tests break down the code you’re testing into small, discrete parts, or “units.” Doing this allows you to test each unit in isolation from the others. This helps you understand how that unit works and interacts with the surrounding code.
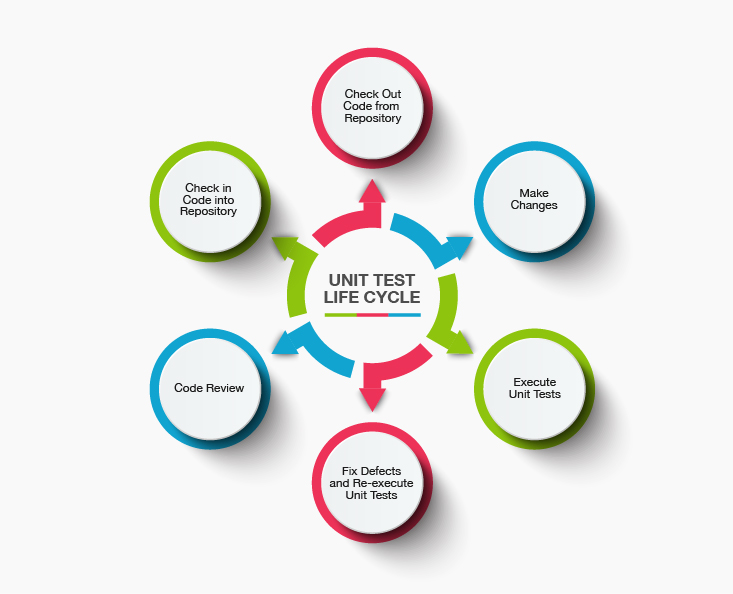
After you’ve written your unit tests, you can run them against your codebase. This will help you to identify any errors or problems that may exist. You can then fix these issues before they cause any real damage.
Types of Unit Testing
There are three unit tests: functional, integration, and sanity.
Functional Testing:
Verifies that each unit of code functions as expected. This testing is done by feeding known inputs into the system and verifying the outputs. For example, you might have a function that takes an integer as input and returns the square of that number. You would test this function by providing different numbers as input and verifying that the output is correct.
Integration testing:
Verifies that units of code interact correctly with one another. This testing is done by combining code units and verifying that the output is correct. For example, you might have a function that takes an integer as input and returns the square of that number. You would test this function by providing different numbers as input and verifying that the output is correct.
Sanity testing:
Sanity testing is a software testing method that confirms that the system behaves as expected under normal conditions. This testing is done to ensure that basic functionality works as expected.
Unit Testing Techniques
Now that you know the basics of this testing let’s look at some techniques you can use.
Mocking:
Mocking is when you create dummy objects for real objects in your code. This allows you to test how your code interacts with other objects without using those other objects.
Stubs:
Stubs are similar to mocks, but they’re typically used to test external API calls. For example, say you’re unit testing some code that makes an HTTP request to an external API. You don’t want to make that HTTP request during your unit test because it’s slow, and it might introduce errors that have nothing to do with your code. Instead, you can create a stub that imitates the API call and returns the data you expect from the real API call.
Data-Driven Testing:
Data-driven testing is where you write your unit tests such that they can be run with different sets of data. This is especially useful if you have a lot of edge cases to test, or if the data you’re working with is too large to hard-code into your unit tests.
Advantages and Disadvantages
Advantages | Disadvantages |
Helps in finding bugs early. The sooner a bug is found, the easier and cheaper it is to fix. | This testing can increase the initial development time. The developer must take the time to write and debug the test cases and write the code itself. |
Helps make the team more efficient in the long run. By this testing, as they code, developers can find and fix bugs more quickly. | This testing can lead to an unnecessary proliferation of test cases. This can lead to increased maintenance time, as the developer must then update and maintain these test cases. |
It makes debugging more manageable, too, since you can pinpoint a bug’s exact location more quickly. | This testing can induce false confidence in the quality of the code. A codebase with many unit tests may give the illusion of being well-tested and free of bugs. However, this is only sometimes the case, as unit tests only test a small portion of the codebase. |
Unit tests can be automated. You can run tests automatically when the code is updated, saving time and human resources. | It can be quite challenging to test the GUI code. |
This testing decreases the total testing cost. By catching bugs early and automating tests, you can save your company time and money in the long run. | This testing can not help catch all the bugs in the application. |
Manual Vs. Automated Unit Testing
Like any other type of testing, unit testing can also be performed manually or automated. In the manual method, developers manually test the code they write. To do so, they might use different inputs and verify if the output is as expected.
Automated testing is another popular way to perform this testing. You can automate using test automation tools or testing frameworks like JUnit, Jest, etc.
How to Write Unit Test Cases
Learning how to write unit test cases is a crucial aspect to know before you learn to create effective test cases.
- Reliable
Create reliable test cases that can run successfully or as expected in any environment. That is, the test result should be the same when run individually or in one go as a whole test suite.
- Readable
Create tests that are easy to understand. That is, even if a test case fails, developers should be able to address the problem easily.
- Fast
Developers run unit tests multiple times to ensure the code is working as expected. So, write unit tests that are environment-independent and faster when run.
- No integration, just unit tests
Unit tests have to be created in a way that is independent and does not involve any external resources for the purpose of testing.
Workflow of Unit Testing
This testing generally follows four steps:
- The first step is creating test cases or figuring out what you want to test and how.
- The second step is writing the code for the tests.
- The third step is running the tests, If the tests pass, the code does what it’s supposed to do, and you can move on. If the tests fail, there’s a bug in the code, and you’ll need to debug it before moving on.
- The fourth step is maintaining the tests. As your code changes, you’ll need to update your tests accordingly. That might mean adding new test cases or modifying existing ones. Read here – Test maintenance
Unit Testing Myth
Myth: It requires more time, and I am always overscheduled. My code is solid and highly precise! I don’t need unit tests.
Myths are false assumptions. And these assumptions will only lead to,
More pressure on you -> You will start writing fewer tests -> Code becomes less stable -> Less productive and accurate you areAnd this will go on in a vicious cycle.
But the truth is this testing improves the development time. Developers think that integration testing will help fix all the errors and do not consider this testing at all. But unit tests will help identify and fix all the simple bugs before even we start integration tests. This will help save time and speed up the development process.
Unit Testing Best Practices
The following are some of the best practices ,
- Unit test cases must be independent. That is, the unit test cases should not be affected even when there are changes in requirements.
- Focus on testing only one module at a time.
- Use consistent and relevant naming conventions for the unit tests.
- Bugs that are detected in this testing have to be fixed before moving to the next phase in the SDLC.
- Follow a test as you code approach. This will help you easily identify and fix bugs.
What Are the Unit Testing Tools?
When it comes to unit testing, a variety of tools can be used to ensure code quality. Popular options include NUnit, XUnit, JUnit, and MSTest, ideal for writing unit tests on programming languages such as Java, JavaScript, and Python. Moreover, the popular Selenium framework is used for automated browser-based testing.
Each tool offers automatic testing execution, parallel test runs, and detailed reporting. They also enable developers to manage their unit tests in test suites and share them across multiple projects. As these tools increase accuracy and productivity regarding unit testing, choosing the right one for your development needs is essential.
Conclusion
In conclusion, unit testing is a crucial part of the software development process that helps ensure the quality and reliability of your code. By writing and running small, isolated tests for individual code units, you can catch errors and bugs early on, improve the maintainability of your code, and build confidence in your work.
To get the most out of this testing, it’s important to write tests that cover a wide range of scenarios and edge cases and to regularly run and update your tests as you make changes to your code.
One way to automate and streamline this testing process is using specialized tools like Testsigma. With Testsigma, you can easily create, execute, and manage your unit tests, saving time and effort while improving the quality of your code. Whether you’re new to this testing or an experienced developer, Testsigma can help you take your testing to the next level.
Frequently Asked Questions:
What Type of Testing is Unit Testing?
Unit testing is a software development process that involves testing individual code units independently from other program portions. It is one of the essential steps in establishing quality control guidelines for a given software project and often results in dramatically fewer errors and defects throughout the programming life cycle.
This testing helps to ensure that all new functions, classes, and modules perform as expected and that changes made to existing code do not break the program. By catching errors at a smaller scale, unit testing minimizes the time and resources necessary for debugging more significant segments of code when problems are found down the line during larger-scale integration tests. Read here – Testing VS Debugging
What Can I Do with Unit Testing?
This testing is great for checking your work as you go. It’s a way to ensure that the code you’re writing does what you want it to.
It’s also a good form of documenting how your code should work when you write unit tests. This can be extremely helpful for other developers who need to work with your code.
Of course, there is a danger zone when it comes to unit testing. If you’re not careful, you can write tests that are too specific to implementing your code. This can make your code more difficult to change in the future.
Common problems with this testing include writing more tests, covering all edge cases, and testing too specific to the implementation.
Can You Use Unit Testing for Security?
Yes, with the shift-left approach, businesses can now create security unit tests early in the SDLC. Earlier, unit tests were created only to test the application’s functional behavior and not the security standards. But unit tests have evolved a lot since then.
By creating security unit tests early in the development cycle, developers can test the application’s security standards and fix the security flaws, if any. It helps create software applications that are highly protected against potential risks or unauthorized access.
Also, conduct peer reviews for security test strategies between developers and application security specialists to help detect edge cases and logical issues, if any.
What Do Unit Tests Look like?
Unit tests are written in code, and they test other codes. They’re usually written in the same language as the code they’re trying, but this isn’t always the case.
Unit tests usually take the form of small functions that call the code you want to test and compare the output to the expected output. If the output matches what was expected, the test passes. If not, the test fails.
Here’s an elementary example in Python:
<!-- wp:paragraph -->
<p>def test_sum():</p>
<!-- /wp:paragraph -->
<!-- wp:paragraph -->
<p>assert sum(1,2) == 3</p>
<!-- /wp:paragraph -->
In this example, we have a function called `test_sum` that takes two values, `1` and `2`, and calls the `sum` function with those values. The expected output is `3`, so we assert that the two values are equal.
If they are, the test passes. If they’re not, the test fails.