The blog post’s title mentions two essential things working in today’s technological world – Javascript and Selenium Webdriver. As an engineer, it would probably be a rare instance if you have never encountered Javascript and Webdriver or heard their names. Currently, there are 1.17 billion websites on the internet today, and the popularity of Javascript is evident that 98% of all websites use Javascript today.
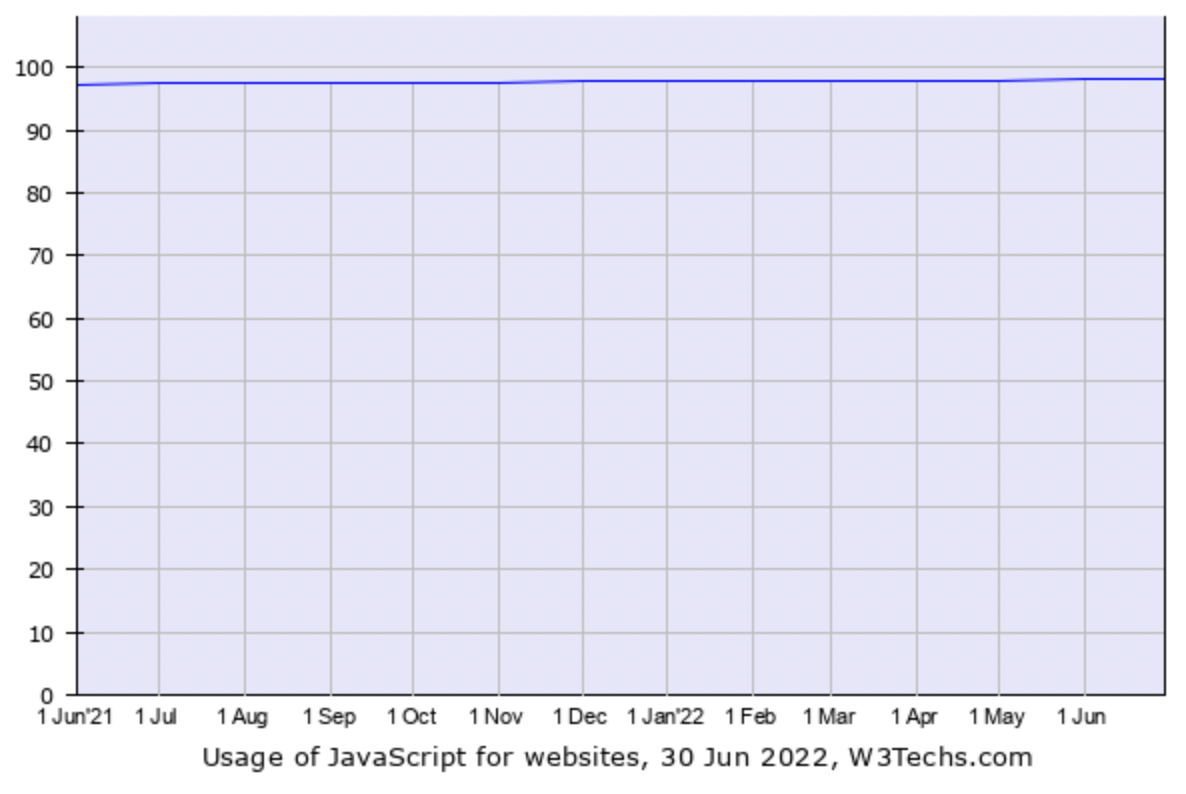
On the other hand, what the developer connects to Javascript is equal to what the tester connects to Selenium. Released in 2004, today, more than 50% of all the testers use Selenium as part of their project. But since Selenium is a framework, it needs the support of a language so that testers can construct test cases in that.
Fortunately, Selenium supports more than 40 languages and frameworks, which gives the tester an added advantage for flexibly choosing their language. But since Javascript is the most popular language in web development, why not start with JS and Selenium and check their relationship with each other?
No matter how hard we try, the vastness of Javascript will stop us from explaining everything related to Selenium and itself. Therefore, in this post, we shall focus on one essential aspect of development and testing – strings in Javascript.
Moreover, we will combine our knowledge of Javascript strings with Selenium and try to execute a demonstration by the end. The highlights of this post may look as follows:
Table Of Contents
- 1 What are Strings in JavaScript?
- 2 Initializing a String in Javascript
- 3 Operations on Strings in Javascript
- 4 Built-in Javascript String Methods
- 5 Javascript String Methods Examples
- 6 Selenium Webdriver and its Footprint
- 7 Installing Selenium on your Personal System
- 8 Is Selenium Enough for Your Needs?
- 9 Why do Developers Favour JavaScript to Write Selenium Test Scripts?
- 10 Best Practices Using Selenium WebDriver with JavaScript
- 11 What do you prefer?
- 12 Frequently Asked Questions (FAQs)
What Are Strings in Javascript?
Before focusing on one programming language and its speciality in strings, let’s simply define a string.
By observing the name “string,” we may think of smaller units of something tied together to make something big. Since programming languages import heavily from real-life practical objects, a string also represents a similar phenomenon in computer science.
A string is a sequence of characters (not a collection like arrays) that make a bigger unit. For example, five characters as “K”, “I”, “T”, “K”, “A”, “T” are independent as written. When they are combined to make a string, they become one unit “KITKAT”.
All the programming languages follow the same upper view concept of a string. Although their construction and usage at the lower level may differ. For example, in Java strings are considered objects but in Javascript, strings are considered primitive data types.
Javascript’s beliefs towards data types have been fairly straightforward and strings could be one of the most used ones among all. The programming language gives us three methods to initialize a string to a variable for further use.
Initializing a String in Javascript
The following three built-in Javascript string methods can be used to initialize a string.
As direct primitive data type:
var myString = “Testsigma”;
As literals
var myString = `Testsigma`;
As an argument to String constructor:
var myString = new String(“Testsigma”);
The third method of using the string constructor is rarely used while programming in Javascript. Also, two different JS string methods that pass the string as an argument in Javascript are as follows:
Method 1:
var myString = new String(“Testsigma”); //Same as defined above
Method 2:
var myString = String(“Testsigma”);
The second call is a function call while the first is the constructor. The difference between these initialization practices is that method 2 converts the passed string to a primitive data type. Whereas method 1 will convert it to a string class object. This will make a difference in the program ahead.
For example, with method 1, a === b will not be equal to true, while in method 2, it will be.
Operations on Strings in Javascript
Strings may have a similar meaning in different programming languages, but operations performed on them differ. This is because of the interpretation of a string by different languages, and so the operations on them differ too. Here we focus on the major operations of the string in Javascript:
Comparing the Strings
In Javascript, unlike other languages like C++, you can directly compare strings through the comparison operator as follows:
var a = “Testsigma”;
var b = “Testsigma”;
if (a === b){
console.log(“The strings are equal”);
} else {
console.log(“The strings are not equal”);
}
Output:

You can also use “<” and “>” operators on the strings. Remember that these operations are case-sensitive. Hence, “Testsigma” and “testsigma” are not equal strings.
Accessing Elements of String
Accessing elements of a string in Javascript is similar to how we access array elements. To achieve this, we use subscripting starting from 0 to a maximum length of the string – 1.
var a = “Testsigma”;
console.log(a[1]);
This will generate output as “e” as it is the second element in the string.
Another method to perform the same task is through the “charAt” function:
var a = “Testsigma”;
console.log(a.charAt(1));
Joining Two Strings
To join two strings together as one, we make use of the assignment operator (+ or +=).
var a = “Testsigma”;
var b = a + ” is a cloud-based tool”;
console.log(b);
Output:
Testsigma is a cloud-based tool.
Built-in Javascript String Methods
Methods | Description |
toUpperCase() | This method converts all the string’s characters into upper case characters. |
toLowerCase() | This method converts all the string’s characters into lower case characters. |
substring() | A part of the string is called a substring. |
replace() | The replace method replaces the string value with the new string as defined in the arguments to the function. It is a helpful method while considering user-based outputs on the web page. |
concat() | The concat() function is used to join two or more strings together as a single string. |
indexOf() | The indexOf method is used to search the occurring index of a particular string in a previously defined string. |
slice() | The slice() method is used to slice or cut a given string. The length of a newly created sliced string depends upon the arguments passed in the function. |
Javascript String Methods Examples
Javascript provides many methods that can be applied to strings and perform complex operations in a single line.
Touppercase()
This method converts all the string’s characters into upper case characters.
var a = “Testsigma”;
console.log(a.toUpperCase());
Output:
TESTSIGMA
When the string is null or undefined, this method does not work.
Tolowercase()
This method converts all the string’s characters into lower case characters.
var a = “Testsigma”;
console.log(a.toLowerCase());
Output:
testsigma
When the string is null or undefined, this method does not work.
Substring()
A part of the string is called a substring. For example, our strings “Testsigma”, “Test”, “T”, “sigma”, “Tes”, and “sig” are all substring. In this string, the characters appear continuously as they do in the original string.
var a = “Testsigma”;
console.log(a.substr(1,4));
Output:
ests
This will provide the substring from 1st index to 4th index.
You can also provide a single number instead as follows:
var a = “Testsigma”;
console.log(a.substr(4));
Output:
sigma
Replace()
The replace method replaces the string value with the new string as defined in the arguments to the function. It is a helpful method while considering user-based outputs on the web page.
var a = “Testsigma is a cloud-based tool”;
var b = a.replace(“cloud-based”, “nlp-based”);
console.log(b);
Output:

Concat()
The concat() function is used to join two or more strings together as a single string.
var a = “Testsigma”;
var b = a.concat(” is a cloud-based tool”);
console.log(b);
Output:
Testsigma is a cloud-based tool
This method is not recommended to use for concatenation of two strings. Please use the assignment operators for the same operation.
Indexof()
The indexOf method is used to search the occurring index of a particular string in a previously defined string.
var a = “Testsigma is a cloud based tool. It is available on the cloud.”;
console.log(a.indexOf(“cloud”));
Output:
15
Here, 15 is the index of the first occurring string “cloud” in the string assigned to the variable “a”.
This function can also pass another argument called “position” as an additional modification. The positional argument defines the minimum index from where JS should look for the string. So, suppose the tester or developer defines the position as 15. In that case, its index value is returned whichever occurrence of the passed string occurs after position 15.
var a = “How much wood would a woodchuck chuck if a woodchuck could chuck wood”;
console.log(a.indexOf(“wood”, 6));
Output:
9
Since the first “wood” appears at the index position 9 which is greater than 6, the number 9 is returned.
While using this argument, we face two types of unwanted situations apart from the above discussed:
1. When the position value is negative: When the defined position value is negative, it is automatically adjusted to 0. So, in this case, it is similar to passing no argument because the first occurrence is returned.
var a = “How much wood would a woodchuck chuck if a woodchuck could chuck wood”;
console.log(a.indexOf(“wood”, -1));
Output:
9
2. When the position value is greater than the last occurring string: In the following snippet, the last “wood” appears at the index value of 65. So, putting the position as 66 would result in an output of -1.
var a = “How much wood would a woodchuck chuck if a woodchuck could chuck wood”;
console.log(a.indexOf(“wood”, 66));
A similar observation can be made when we pass a position value longer than the string length. The value returned is -1.
Please note that this argument is optional in the method.
Slice()
The slice() method is used to slice or cut a given string. The length of a newly created sliced string depends upon the arguments passed in the function.
The method takes two arguments called starting index and ending index. However, if only one value is passed, it is considered the starting index value.
Similar to the indexOf function, we also face certain situations in the slice() method. These are summarized as follows:
Starting index situations:
1. When starting index is not passed: If neither the starting index nor the ending index is passed, the resultant string is the original string.
var a = “Testsigma”;
console.log(a.slice());
Output:
Testsigma
2. When the starting index is passed as negative: In such a case, the starting index value is taken as string length + starting index. For example, if a string of length 7 is sliced with the starting index as -2, the slicing will begin from 7 – 2 i.e. 5th index value. If the ending index value does not pass, the string is sliced to the end of the string.
var a = “Testsigma”;
console.log(a.slice(-2));
Output:
ma
3. When the starting index is passed as more than string length: In such a case, an empty string is returned without any errors.
var a = “Testsigma”;
console.log(a.slice(11));
Output:
“”
Ending index situations:
1. When the ending index is not passed: In such a case, the ending index becomes the length of the string. Therefore, the sliced string returned is from the starting index to the end of the string.
var a = “Testsigma”;
console.log(a.slice(2));
Output:
stigma
2. When the ending index is negative: In such a case, the final ending value calculated is as “string length + ending index”. For example, if the ending index value passes as -2 to a string of length 7 then the final ending index value is calculated as 7 – 2 i.e. till the 5th index value.
var a = “Testsigma”;
console.log(a.slice(0, -2));
Output:
Testsig
3. When the ending index value is greater than the string length: In such a case, the ending index is considered as the string length. So the sliced string is from the starting index to the end of the string.
var a = “Testsigma”;
console.log(a.slice(0, 20));
Output:
Testsigma
Apart from these popular methods used on Javascript strings, you may find a lot more methods described in the string library. Although, these methods would suffice a lot of your requirements.
Selenium Webdriver and Its Footprint
Completing the basic overview of Javascript, the next part of our post is around Selenium. As a tester, either manual, automation, QA, or anything, you will always come across the popularity of Selenium and the benefits it brings with it.
Selenium is a test automation tool used to automate the tasks related to the browsers using browser drivers. In 2022, the state of test automation survey found out that more than 64% of QAs and other automation testers prefer Selenium for their automation tasks. It is software released 18 years ago but is still popular in test automation.

This data is not surprising as Selenium has been one of the most popular tools since its release in 2004. Over the years, Selenium has also become much more powerful and has introduced features other than “browser task automation”. The top-level offerings from Selenium may look as follows:
1. Selenium IDE: The integrated development environment offered by Selenium offers smooth integration with Selenium tests. Through the IDE, we can implement popular “record and replay” tests initiated by Selenium and could be regarded as the foundation for codeless testing. With Selenium IDE, you can record your scripts and then run them as many times (including editing) later.

Source – Selenium IDE for Chrome (Google Chrome Extension)
2. Selenium Client API: The Selenium Client API helps in calling functions available in Selenium and runs on a browser with many different languages.
3. Selenium RC: The Selenium RC (abbreviated for Remote Control) helps communicate between HTTP requests and the server. It is written in the Java language and is the reason that makes it possible to write Selenium commands in any programming language. It was released in the initial versions of Selenium which was later enrolled in the Selenium Webdriver.
4. Selenium Webdriver: The Selenium Webdriver is probably the most popular and used part of the Selenium suite. The WebDriver started as a successor to Selenium RC and is used to send commands to the browser driver. The commands are written as the testing scripts. These work as the actions that are required to be performed on the browser. Once run, the browser driver instance is launched, and test results are displayed to the tester.
5. Selenium Grid: The Selenium Grid is a server-based infrastructure that may look as follows:
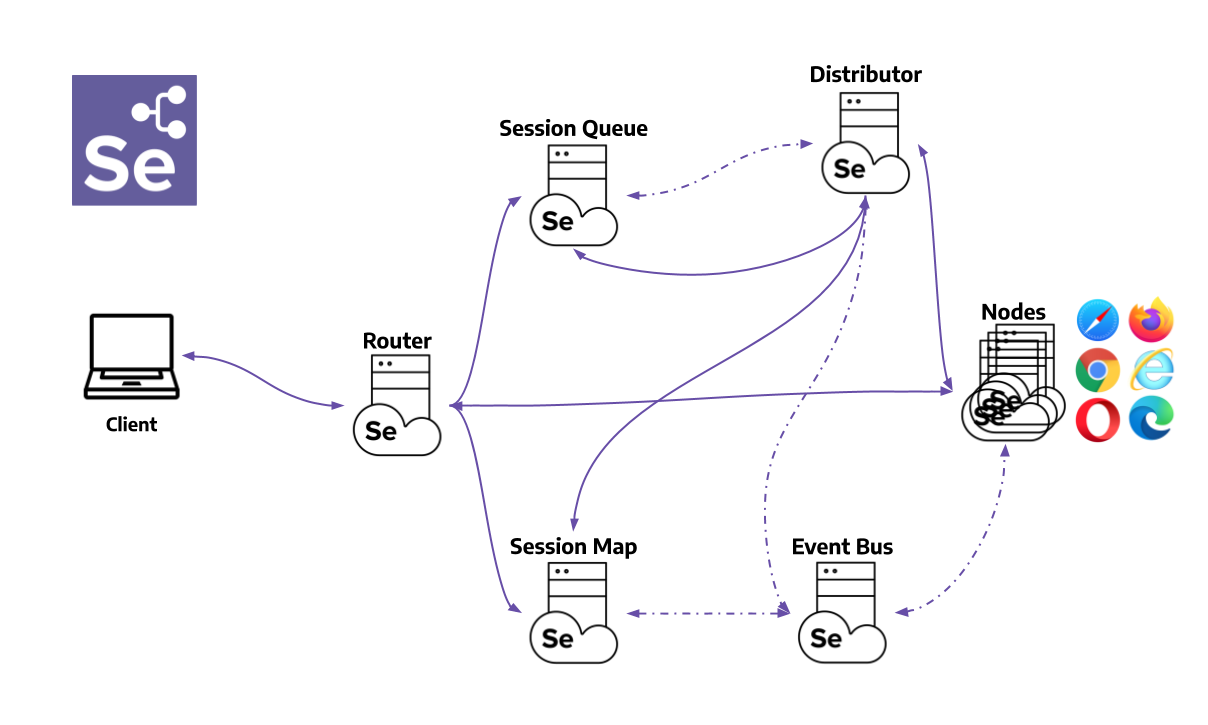
In this arrangement, the client sends a request to the hub that contains the information about the connected nodes (or server). The request is then forwarded to the appropriate node available on the cloud to perform the action.
Apart from this, you may also find a bit of smaller components related to Selenium just like Javascript. Since it is an actively developed open-source project, discussing all the components would not be possible in a single post. We recommend going through Selenium’s official website once to get a brief introduction to its smaller components.
Over the years, Selenium has adopted to a long list of programming languages. These include Javascript, Java, Python, and a lot more. In this post, we focus on the Javascript strings part of the Selenium. For this, we need to install Selenium and Javascript dependency for it on our personal system.
Installing Selenium on Your Personal System
Selenium can be downloaded from its official website for any system you want to run the scripts for. Later, you need to add the libraries depending on your IDE. If IntelliJ or Eclipse, you must add the libs in the appropriate columns. For simpler IDEs like Atom, adding the global variables to the system environment also works.
Once the installation and setup are done, we need to download the drivers for the browsers we will run the tests on. For example, if you want to run on the Chrome browser, you need to download the chrome driver on the system. This can be achieved by its official website and adding the same to the system path variable.
Next, open the project folder and add a javascript file in which we will write the test cases.
Generally, test files are created inside the “test” folder, which is often pre-defined in the project folder. It is also located in the project directory, but the file can work from anywhere inside the project tree.
Add the following line in the JS file:
const webdriver = require(‘selenium-webdriver’),
By = webdriver.By,
until = webdriver.until;
const driver = new webdriver.Builder()
.forBrowser(‘firefox’)
.build();
driver.get(‘http://www.google.com’);
driver.getTitle().then(function(title) {
if(title === ‘webdriver – Google Search’) {
/* Do something with JS strings */
console.log(‘Test passed’);
} else {
console.log(‘Test failed’);
}
driver.quit();
});
Here, we have used the firefox web driver. In the above snippet, you can see the “Do something with JS strings” line. Here, you can use JS strings operations to perform on the title you retrieve from the browser tab.
Is Selenium Enough for Your Needs?
Selenium is an excellent tool that can automate almost everything you want. However, is it enough for your needs considering scalability and industry-sized code bases?
A direct answer is yes. Selenium is strong and capable. But, it is not as straightforward as we wrote the simple code above. With scalability comes complexity, for which you may have to invest time and hire experts (with higher salaries) to manage such infrastructure. So, can we achieve the same (or better) efficiency using another tool than Selenium?
Testsigma is a tool/platform working in the same test automation domain and provides a much easier method of testing the application. The main benefit of using Testsigma is its usage of the English language for writing test cases which uses natural language processing at the backend. These types of test cases are easier to understand and maintain than scripted ones as done in Selenium.

On top of it, Selenium also comes with integrated artificial intelligence technology that can self-heal the test cases, detect failures upfront, and adjust test cases according to the changes in the software. This might not be possible with Selenium.
Since there are too many parameters to consider, we can summarise them in the following table as a comparison of Selenium and Testsigma:
Parameter | Selenium | Testsigma |
Setup costs and time | High | Low |
Test development time | High | Low (10x faster) |
Execution time | High | Low |
Maintenance time | High | Low (70% lower) |
Response to changes | Slow | High (AI-enabled) |
Achieving test coverage time | High | Low |
ROI time | High | Low (Since Day 1 due to low initial costs) |
Team | Programming and automation experts. | Anyone can perform automation. |
Integration with other tools | Hard | Extremely easy |
Support | Forums and communities | Dedicated team available 24 X 7 |
Observing the above table, it is evident that Testsigma is easier to operate and lets you write test cases that run more efficiently within time limits. This will help you release the software timely, primarily if agile or in-sprint methods are used in your organization.
Why Do Developers Favour Javascript to Write Selenium Test Scripts?
When there are multiple languages you can choose from to write test scripts in Selenium, for what reasons do developers prefer JavaScript? We have the answers:
- JavaScript is a well-known language that many professionals have the skills for
- The combination of Selenium WebDriver with JavaScript is suitable for carrying out UI testing
- JavaScript offers improved security and receives support from a large community of developers
- It is a widely popular option for developing web applications, as a large group of web applications using the MEAN—MongoDB, Express.js, AngularJS, and Node.js—stack for development
- JavaScript offers a huge library of plugins, APIs, and frameworks for testers to easily perform testing scenarios
Best Practices Using Selenium Webdriver with Javascript
Here are some of the best practices for using Selenium WebDriver with JavaScript:
- Use the right locators: The Selenium framework interacts with the browser. So, it is essential to use the right web element locators to better navigate the objects with the Document Object Model (DOM).
- Perform data-driven testing: Ensure to go for data-driven testing as it will help you accurately and readily perform functional testing.
- Implement page object model: Reduce redundancy and improve maintenance by the implementation of PageObjects. Here, each web page is considered a class file, and every class file corresponds to web elements. It helps eliminate code duplication.
- Go for the right selector order: For faster testing, the right selector order is fairly necessary. Understand how to handle web elements in Selenium.
What Do You Prefer?
After learning about the most popular development language and one of the most popular test automation tools, we are here to conclude our learnings. In Javascript, you would often use the string operations to operate on the data you fetch from the scripts. Since assertions work on comparing expected and actual results, the methods shared in this post will prove to be helpful in your following projects.
On the other hand, Selenium can automate the browsers and perform actions on them similar to what a user would do. This is helpful when we need to test cases on many browsers simultaneously. Combine this with Javascript, and we get the perfect combination.
With this, we hope you try Testsigma once to compare your experience with Selenium and settle on what feels best for you. If you have various tools in the comment section. Thank you for your time.
Frequently Asked Questions (faqs)
How to Input String in Javascript?
To input a string in JavaScript, you can use the prompt() function. The parameter of this function is the string value that users want to display or use in the program.
What is a String in Javascript, and What Are Its Functions?
In JavaScript, a string is a series of characters that make a bigger unit. It has multiple functions that manipulate the original string. We have discussed all the string functions in this blog.
Can You Use Selenium with Javascript?
Selenium is compatible with JavaScript. It is an open-source automation testing tool that supports plenty of other languages, including JavaScript, to perform testing. Some of them are C#, Java, Perl, and Ruby.
Why is Javascript Used in Selenium?
JavaScript with Selenium helps to enhance the security of the test scripts without compromising the overall results. You can read more about why JavaScript is useful for Selenium in the above sections.