Selenium is a test automation tool for automating user actions on a web browser. In this guide, we will walk you through the process of using Selenium to click on different types of elements.
Whether you’re a beginner or an experienced user, this guide will provide you with the knowledge and skills to effectively use Selenium for clicking on various elements on web pages. So, let’s learn how to master the Selenium click function!
Table Of Contents
What is the Selenium Click Command?
The Selenium Click Command is a way to simulate a mouse click in Selenium WebDriver. It interacts with buttons, links, and other interactive elements on a web page. The click() method is a member of the WebElement interface and performs a click action on a specific web element.
Here’s an example in Java:
1
2// Import the WebDriver package
3import org.openqa.selenium.WebDriver;
4import org.openqa.selenium.firefox.FirefoxDriver;
5import org.openqa.selenium.By;
6
7public class SeleniumClick {
8 public static void main(String[] args) {
9 // Create a new instance of the Firefox driver
10 WebDriver driver = new FirefoxDriver();
11
12 // Navigate to the webpage you want to interact with
13 driver.get(“http://www.example.com”);
14
15 // Locate the element you want to click on
16 WebElement element = driver.findElement(By.id(“click-me”));
17
18 // Perform the click action on the element
19 element.click();
20
21 // Close the browser
22 driver.quit();
23 }
24}
25
Here, we import the WebDriver and By classes from the Selenium library in this example. Then, we create an instance of the Firefox driver, which allows us to control the Firefox browser. Next, we use the get function to navigate the webpage we want to interact with.
After that, we use the findElement method and the By.id locator to locate the element on the page we want to click. Finally, we use the click method to perform a mouse click on the element.
How to Use the Selenium Click Command?
How to Perform a Right Click in Selenium?
The right-click action in Selenium WebDriver can be performed using the Actions class. The context_click() method provided by the Actions class is used to perform this operation. This method is also known as a right-click or context-click.
You can perform a right-click in Selenium using the context_click() method of the ActionChains class. The ActionChains class allows you to simulate mouse and keyboard actions in Selenium.
Here is an example of how you can use the context_click() method to right-click on a button with a certain id using Java and the Selenium WebDriver:
1
2import org.openqa.selenium.By;
3import org.openqa.selenium.WebDriver;
4import org.openqa.selenium.WebElement;
5import org.openqa.selenium.firefox.FirefoxDriver;
6import org.openqa.selenium.interactions.Actions;
7
8public class Main {
9public static void main(String[] args) {
10// Start a webdriver and navigate to a webpage
11WebDriver driver = new FirefoxDriver();
12driver.get(“http://example.com”);
13
14 // Find the button element
15 WebElement button = driver.findElement(By.id(“my-button”));
16
17 // Create an Actions object and right-click on the button
18 Actions actions = new Actions(driver);
19 actions.moveToElement(button).contextClick().build().perform();
20}
21
22}
23
This will simulate a mouse hover and right-click action.
Here is an example of how you can use the context_click() method with a different locator method, xpath, in Java:
1
2import org.openqa.selenium.By;
3import org.openqa.selenium.WebDriver;
4import org.openqa.selenium.WebElement;
5import org.openqa.selenium.firefox.FirefoxDriver;
6import org.openqa.selenium.interactions.Actions;
7
8public class Main {
9public static void main(String[] args) {
10// Start a webdriver and navigate to a webpage
11WebDriver driver = new FirefoxDriver();
12driver.get(“http://example.com”);
13
14 // Find the element using xpath
15 WebElement elem = driver.findElement(By.xpath(“//xpath”));
16
17 // Create an Actions object and right-click on the element
18 Actions actions = new Actions(driver);
19 actions.moveToElement(elem).contextClick().build().perform();
20}
21
22}
23
It is important to note that before using the context_click() method, you must first locate the element using any of the locator methods available, such as By.id, By.name, By.xpath, and many others. Ensure that the element is visible and enabled before performing any actions.
Read here: XPath vs CSS locator: Key Differences
How to Perform a Left Click in Selenium?
A left click is the primary option for everyone using a computer. In Selenium, to perform a left click, you need to first identify and locate the element. After this step is complete, you can execute the left-click operation using the click() method.
Suppose you want to click on the Schedule a Demo option on Testsigma homepage.
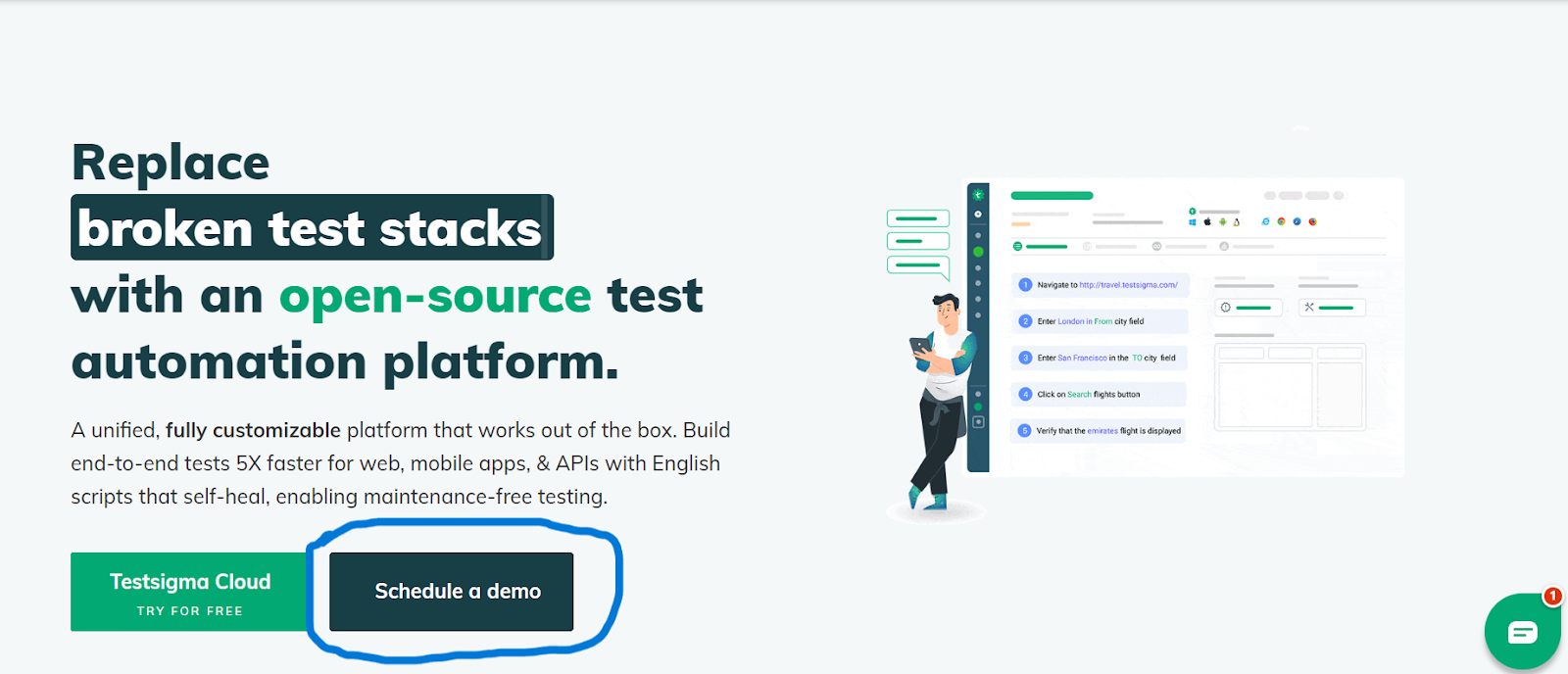
The code for the above operation would be:
driver.get("https://testsigma.com/");
driver.findElement(By.id("")).click(); //id field will as per the code
This navigates to the Testsigma website, locates the ‘Schedule a demo’ button using its “id” and performs the left-click action.
Performing a Double Click in Selenium
You can perform a double click in Selenium by using the double_click() method of the ActionChains class.
The ActionChains class allows you to simulate mouse and keyboard actions in Selenium.
Here’s an example of how you can use the doubleClick() method to double-click on a button with a certain ID using Java:
1
2import org.openqa.selenium.WebDriver;
3import org.openqa.selenium.firefox.FirefoxDriver;
4import org.openqa.selenium.interactions.Actions;
5import org.openqa.selenium.WebElement;
6
7public class Main {
8public static void main(String[] args) {
9// Start a webdriver and navigate to a webpage
10WebDriver driver = new FirefoxDriver();
11driver.get(“http://example.com”);
12
13// Find the button element
14WebElement button = driver.findElement(By.id(“my-button”));
15
16// Create an Actions object and double click on the button
17Actions actions = new Actions(driver);
18actions.moveToElement(button);
19actions.doubleClick();
20actions.perform();
21}
22}
23
This will simulate a mouse hover and double-click action in the web page.
Here’s an example of how you can use the double_click() method to double-click on an element with a specific xpath using Java and Selenium:
1
2import org.openqa.selenium.By;
3import org.openqa.selenium.WebDriver;
4import org.openqa.selenium.WebElement;
5import org.openqa.selenium.firefox.FirefoxDriver;
6import org.openqa.selenium.interactions.Actions;
7
8public class Main {
9public static void main(String[] args) {
10// Start a webdriver and navigate to a webpage
11WebDriver driver = new FirefoxDriver();
12driver.get(“http://example.com”);
13
14// Find the element by xpath
15WebElement elem = driver.findElement(By.xpath(“//xpath”));
16
17// Create an Actions object and double-click on the element
18Actions actions = new Actions(driver);
19actions.moveToElement(elem).doubleClick().build().perform();
20
21}
22}
23
It is important to keep in mind that before using the double_click() method, you must first locate the element using any of the available locator methods, such as findElement(By.id), findElement(By.name), findElement(By.xpath), etc.
Additionally, make sure the element is visible and enabled before performing any actions. It is recommended to use a try-catch block to handle any exceptions that may occur while interacting with the elements.
Read more: Find Element by XPath
Commands to Perform Selenium Click
There are several ways to perform a click action in Selenium, depending on the type of element you are trying to interact with. Here are a few examples:
1. Using the Click() Method
This is the most common way to perform a click action in Selenium. Once you have located the element you want to interact with, you can use the click() method to trigger a click event.
Here’s an example of how you can use the ‘click()’ method to click on a button with a certain id Java using Selenium WebDriver:
1
2WebDriver driver = new FirefoxDriver();
3driver.get(“https://www.example.com”);
4
5WebElement button = driver.findElement(By.id(“my-button”));
6button.click();
7
In this example, the FirefoxDriver object initializes the web driver, allowing you to interact with a website in a Firefox browser. The get method is then used to navigate to a specific URL.
Next, the findElement method locates the button element on the page. The By.id locator is used to locate the element by its ID attribute, with the ID value passed as an argument.
Finally, the click method is called on the WebElement object to simulate a mouse click on the button. This will trigger the action associated with the button, such as submitting a form or navigating to a new page.
Another way of clicking is by calling execute_script() and passing the javascript command:
1
2WebDriver driver = new FirefoxDriver();
3driver.get(“https://www.example.com”);
4
5WebElement button = driver.findElement(By.id(“my-button”));
6((JavascriptExecutor) driver).executeScript(“arguments[0].click();”, button);
7
You can also click on an element using the click method of the Actions class, which allows you to simulate mouse and keyboard actions in Selenium WebDriver:
1
2WebDriver driver = new FirefoxDriver();
3driver.get(“https://www.example.com”);
4
5WebElement button = driver.findElement(By.id(“my-button”));
6
7Actions actions = new Actions(driver);
8actions.moveToElement(button).click().perform();
9
This will simulate a mouse hover and click action on the button element.
2. Using the Send_keys() Method
The send_keys() method in Selenium is used to simulate text typing into an input field or text area on a webpage. This method is commonly used when interacting with forms or text fields.
Here’s an example of how to use the send_keys() method in Selenium to type text into an input field:
1
2import org.openqa.selenium.By;
3import org.openqa.selenium.WebDriver;
4import org.openqa.selenium.firefox.FirefoxDriver;
5
6public class Example {
7 public static void main(String[] args) {
8 // create a new instance of the Firefox driver
9 WebDriver driver = new FirefoxDriver();
10
11 // navigate to the webpage you want to interact with
12 driver.get(“http://www.example.com”);
13
14 // locate the input field you want to type into
15 driver.findElement(By.id(“input-field”)).sendKeys(“Hello, World!”);
16 }
17}
18
In this example, we first import the necessary classes from the Selenium library. Next, we create an instance of the Firefox driver, which allows us to control the Firefox browser.
Then, we use the get() method to navigate to the webpage we want to interact with. After that, we use the findElement() method along with the By.id() locator strategy to locate the input field on the page that we want to type into.
Finally, we use the sendKeys() method to type the text “Hello, World!” into the input field.
3. Using the Actionchains Class
The ActionChains class in Selenium is used to automate complex user interactions such as mouse movements, clicks, and keyboard events. This class allows you to create a sequence of actions performed one after the other.
Here’s an example of how to use the ActionChains class in Selenium to perform a double-click action on an element:
1
2import org.openqa.selenium.By;
3import org.openqa.selenium.WebDriver;
4import org.openqa.selenium.firefox.FirefoxDriver;
5import org.openqa.selenium.interactions.Actions;
6
7public class Example {
8 public static void main(String[] args) {
9 // create a new instance of the Firefox driver
10 WebDriver driver = new FirefoxDriver();
11
12 // navigate to the webpage you want to interact with
13 driver.get(“http://www.example.com”);
14
15 // locate the element you want to double-click on
16 driver.findElement(By.id(“double-click-me”));
17
18 // create an Actions object
19 Actions actions = new Actions(driver);
20
21 // perform the double-click action on the element
22 actions.doubleClick().perform();
23 }
24}
25
It’s important to ensure that the element is visible and interactable before performing actions. It’s useful for elements that are difficult to interact with using standard Selenium WebDriver methods.
4. Using Javascript
Selenium WebDriver allows you to execute JavaScript code in the browser using the execute_script() method. This can be useful for performing actions not natively supported by Selenium or bypassing limitations of the standard Selenium WebDriver methods.
Here’s an example of how to use the execute_script() method in Selenium to click on an element:
1
2# create a new instance of the Firefox driver
3driver = webdriver.Firefox()
4
5# navigate to the webpage you want to interact with
6driver.get(“http://www.example.com”)
7
8# locate the element you want to click on
9element = driver.find_element_by_id(“submit-button”)
10
11# click the element using JavaScript
12driver.execute_script(“arguments[0].click();”, element)
13
Selenium’s execute_script method allows you to execute JavaScript code in the browser. It can be useful to perform actions not natively supported or bypass the limitations of standard Selenium WebDriver methods.
Remember to check if the element is visible and interactable before performing actions.
Advanced Click Techniques: Sending a Right-click Mouse Event
In addition to the context_click() method, Selenium provides a way to send a right-click mouse event to an element using the send_keys() method in conjunction with the Keys.CONTROL key.
Here’s an example of how you can use the send_keys() method to send a right-click mouse event to an element:
1
2import org.openqa.selenium.WebDriver;
3import org.openqa.selenium.firefox.FirefoxDriver;
4import org.openqa.selenium.Keys;
5import org.openqa.selenium.WebElement;
6import org.openqa.selenium.interactions.Actions;
7
8// Start a webdriver and navigate to a webpage
9WebDriver driver = new FirefoxDriver();
10driver.get(“http://example.com”);
11
12// Find the element
13WebElement elem = driver.findElement(By.xpath(“//xpath”));
14
15// Send a right-click mouse event to the element
16elem.sendKeys(Keys.CONTROL + Keys.RETURN);
17
18// Another alternative way of doing this is using ActionChains:
19Actions actions = new Actions(driver);
20
21// Move to element location
22actions.moveToElement(elem);
23
24// Right click on element
25actions.contextClick(elem).perform();
26
In some cases, the elements might be hidden and not visible, and you can either use JavascriptExecutor to unhide the elements or use the Keys.CONTROL + Keys.RETURN to send a right-click event to the element.
This technique may not work on some of the websites and applications. In those scenarios, you have to use JavascriptExecutor.
It’s always a good practice to use try-catch blocks to catch any exceptions while interacting with the elements.
Summary
Whether you’re using Selenium for web scraping, testing, or any other purpose – Clicking on an element is a basic action. Thus, it is important to learn how to use Selenium to automate the click action.
We hope this guide has provided you with a solid foundation for using Selenium to automate click actions on elements.
But, using Selenium also means you need to be an expert in coding in one of the supported programming languages. Also, using code to automate your actions can take more time.
Testsigma, is an Gen AI-powered, no-code test automation tool that is built to solve these problems. Being, no code, it lets you automate all these actions without learning to code. And, it takes much less time too!
Frequently Asked Questions:
How Do You Trigger a Click Element?
To trigger a click on an element in web automation, follow below steps:
- Locate the element first (The element can be located using various locator strategies such as ID, class name, name, xpath, etc. )
- Use the click() method to simulate a mouse click on that element.
Example code:
1
2WebDriver driver = new FirefoxDriver();
3driver.get(“https://www.example.com”);
4
5WebElement button = driver.findElement(By.id(“my-button”));
6button.click();
7
How to Click Using Xpath in Selenium?
Use the find_element_by_xpath() method of the WebDriver class and then call the click() method of the WebElement class.
It’s also a good idea to test your XPath expressions to make sure they correctly locate the desired element before using them in your test code.
What is the Method Used to Perform Clicks?
In Selenium, the click() method performs a click action on a web element. The click() method is a part of the WebElement class in Selenium.
This method is used after an element is located by any available locator strategies like XPath, id, name, class name, and many others.