Listeners in Selenium – A Guide to Enhance Test Automation
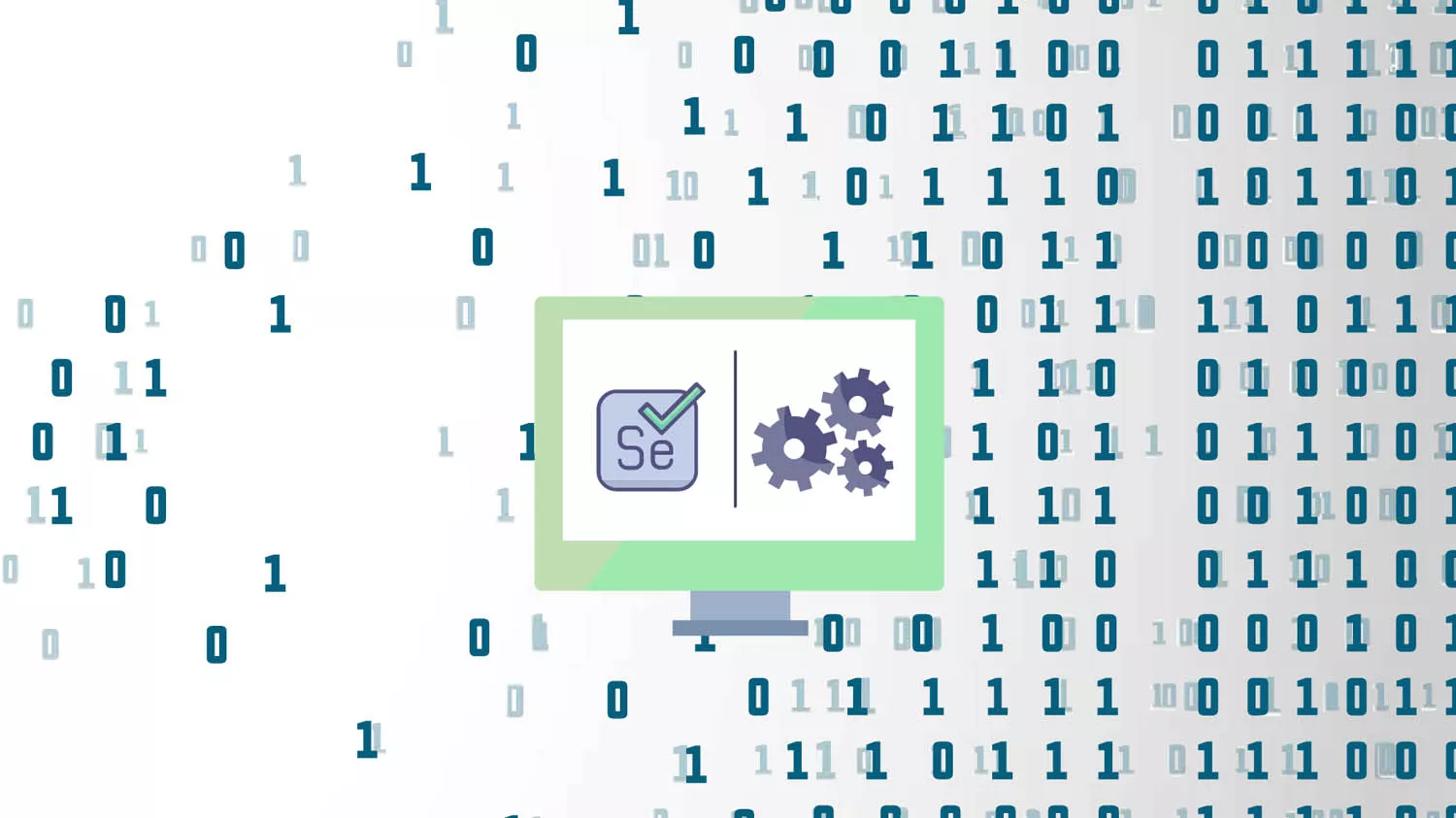
Listeners in Selenium are classes that implement specific interfaces in order to track events that occur during the execution of Selenium tests. You can use them to perform additional actions or log information when specific events happen, such as a test case starting or ending, or a test step passing or failing. Using listeners, developers can add extra functionality and customization to their Selenium tests without modifying the test code.
In this article, we will understand the concept of listeners in Selenium. We will walk you through the two types of listeners in Selenium – TestNG and WebDriver listeners. We will also see how to implement these listeners along with their use cases.
Table Of Contents
- 1 What are Listeners in Selenium?
- 2 Types of Listeners in Selenium
- 3 What are WebDriver Listeners in Selenium?
- 4 What are TestNG listeners in Selenium?
- 5 Prerequisites to use listeners in Selenium using Java
- 6 Implementing Listeners in Selenium WebDriver Script
- 7 Steps to create a TestNG Listener
- 8 Use of listeners in Selenium for multiple classes
- 9 Conclusion
- 10 Frequently Asked Questions
What are Listeners in Selenium?
In Selenium, a listener is an object that “listens” for certain events to occur during the execution of a test script, such as the start or completion of a test case, or the detection of an error. Hence, when an event of interest occurs, the listener can perform a specific action, such as logging information about the event or taking a screenshot.
Here are a few examples of how listeners can be used in Selenium:
- Screenshot on failure: You can use a listener to take a screenshot of the browser window when a test case fails. This can be useful for debugging and identifying the cause of the failure.
- Logging: You can also use them to log information about the test execution, such as the start and end time of each test case, and the test case status (pass/fail). This can be useful for generating detailed test execution reports.
- Email notification: They can be used to send an email notification when a test execution completes. This can be useful for alerting the development team of the test execution results.
- Performance Metrics: A listener can also be used to measure and record the execution time of each test method. It can be used to log test-wise or suite-wise performance metrics, which will be helpful in identifying performance bottlenecks.
As a result, we can use listeners to add additional functionality to a test script without modifying it, making it easier to maintain and extend the test suite over time.
Types of Listeners in Selenium
In Selenium, there are two types of listeners.
- TestNG listeners.
- WebDriver listeners.
We use TestNG listeners to perform operations before and after a test method is executed. On the other hand, we use WebDriver listeners to listen to WebDriver events like navigating to a new URL and clicking an element.
It’s worth noting that there are several built-in listeners which are provided by Selenium. In addition to this, developers can also create their own custom listeners by implementing the interfaces or classes and registering them to listen to the events. Let’s have a look at both these types of listeners in detail.
What are WebDriver Listeners in Selenium?
WebDriver listeners in Selenium are event listeners that allow you to listen to events emitted by the WebDriver. Consequently, these listeners provide an extra layer of functionality. This allows you to capture and process events, such as navigating to a new URL or clicking on an element.
Built-in WebDriver Listeners
Selenium provides several built-in WebDriver listeners. We can use them to capture and process events emitted by the WebDriver. These include:
- WebDriverEventListener: This is an interface that contains methods for listening to WebDriver events.
- AbstractWebDriverEventListener: It is an abstract class that you can subclass to implement the WebDriverEventListener interface.
- EventFiringWebDriver: This class wraps up a WebDriver instance and enables the firing of WebDriver events.
- WebDriverListenerManager: A class that manages the registered listeners and notifies them of the events.
Use Cases of WebDriver Listeners
WebDriver listeners are particularly useful in cases where you need to capture and process events emitted by the WebDriver. Some common use cases include:
- Taking screenshots of test failure.
- Logging test results.
- Capturing performance metrics.
- Analyzing browser logs.
In summary, WebDriver listeners in Selenium allow you to capture and process events emitted by the WebDriver. These listeners provide an extra layer of functionality, making it easier to capture and process events in your tests.
What are TestNG listeners in Selenium?
As discussed earlier, we use TestNG listeners in Selenium to perform operations before and after we execute a test method. We can provide additional functionality to TestNG tests, such as capturing screenshots or logging test results. Let’s have a look at built-in TestNG listeners in Selenium and their use cases.
Built-in TestNG Listeners
As discussed, we have several built-in TestNG listeners in Selenium. These can be used to perform operations before and after a test method is executed. These include:
- IInvokedMethodListener: This listener is invoked before and after every test, the method is invoked.
- IAnnotationTransformer: It is used to modify test method annotations.
- IMethodInterceptor: This listener is used to add an interceptor to the test method invocation.
- IHookable: It is used to execute any code before and after a test method, test configuration method, or suite.
- IConfigurationListener: This listener is invoked before and after a configuration, and the method is invoked.
- IExecutionListener: It is invoked at the beginning and end of a test run.
- ISuiteListener: This listener is invoked before and after a suite is run.
- ITestListener: It is invoked before and after a test is run and on test success or failure.
Use Cases of TestNG Listeners
TestNG listeners are particularly useful in cases where you need to perform operations before and after a test method is executed. Some common use cases include:
- Taking screenshots of test failure.
- Logging test results.
- Capturing performance metrics.
- Analyzing browser logs.
- Interceptors for test methods.
In summary, TestNG listeners in Selenium allow you to perform operations before and after a test method is executed. Furthermore, with the use of these listeners in Selenium, it’s easier to add additional functionalities to your test cases.
Prerequisites to use listeners in Selenium using Java
Here are a few steps to get you started.
- Download the latest version of Maven from the Apache Maven website (https://maven.apache.org/download.cgi)
- Extract the downloaded file to the location where you want to install Maven (e.g. C:\maven)
- Add the Maven bin directory to the system path environment variable. To do this, open the System Properties window, click on the Advanced tab, and then click on Environment Variables. Under System Variables, scroll down and find the Path variable, then click on Edit. Add the path to the Maven bin directory (e.g. C:\maven\bin) to the end of the Variable value.
- Open a command prompt and type “mvn -version” to check whether maven is installed successfully and check the version.
- Create a new folder for your Selenium WebDriver project and navigate to it in the command prompt.
- Create a new file called “pom.xml” in the project directory, and copy the following code into it:
Make sure to replace “LATEST_VERSION” with the latest version of Selenium and TestNG.

- Run the command “mvn clean install” in the project directory to download the Selenium and TestNG dependencies.
- Once the dependencies are downloaded, you can create your listener class and test classes, and run the tests using TestNG.

Please note that this is just an example of pom.xml file. You can add more dependencies or plugins as per your project requirements.
Implementing Listeners in Selenium WebDriver Script
We can implement Listeners in Selenium WebDriver scripts in several ways. Here’s an example of how to implement a WebDriver listener in Java:
First, create a class that implements the WebDriverEventListener interface. This class should override the methods for the events that you want to listen to.
Code Example

Creating Web Drivers
First, you will have to install an executable webDriver in your system. You can download the latest version of ChromeDriver from the ChromeDriver website and make sure it is compatible with your version of Chrome.
Next, create an instance of the EventFiringWebDriver class, passing the original WebDriver instance as a parameter to the constructor.
Register the listener with the EventFiringWebDriver instance by calling the register() method and passing an instance of your listener class as a parameter.
Unregister the listener to stop listening to the events
In this example, we’ve created a listener class called “MyListener” that implements the WebDriverEventListener interface. This interface makes it seamless for us to use WebDriver listeners in Selenium. The class has overridden methods before and after navigating to any URL.
Then we created an instance of the EventFiringWebDriver, passed an instance of Chrome driver as a parameter, and registered the listener with it. Then by using this instance of EventFiringWebDriver, we are navigating to google.com, which will invoke the overridden methods of the listener class. Finally, it also shows how to unregister the listener once it’s not needed anymore.
Moreover, it’s worth noting that you can add multiple listeners, and you would need to unregister each of them individually. Also, there are other ways too. You can use a WebDriverListenerManager to add and remove listeners instead of EventFiringWebDriver.
Complete Code

Steps to create a TestNG Listener
Creating TestNG listeners in Selenium is a powerful way to add extra functionality to your tests. Here’s an example of how to create a TestNG listener in Java:
Code Example
We create a class that implements the ITestListener interface. This class will override the methods for the events that we want to listen for:

We can add the listener class to our TestNG test class by annotating it with the @Listeners annotation, this way you don’t need to add the listener class to the testng.xml file:

In this example, the TestNG listener class “MyListener” is implementing all the overridden methods of ITestListener interface. Also, there is a test class TestClass with two test methods test1 and test2, that is annotated with @Listeners(MyListener.class). When you run the test class, the methods of the listener class will be invoked at the appropriate time during the test execution.
Using an XML file to define Listeners and Test Classes
You can also add it using an XML file.
In this example, we have added the listener class to the testng.xml file by adding it to the listeners tag and then added the test class inside the <classes> tag. When you run this testng.xml file, it will look for the listener and use it during the execution of the test class. You can also use multiple listeners by adding them to the listeners tag or the @Listeners annotation, in the same way. You can also use the <class> tag multiple times to specify multiple classes for testing.
As a reminder, you need to have testng.xml in the root of your project and should execute it with the command: mvn test -Dtest=testng.xml.You can also run the testng.xml file via the command-line interface, by using the command: java org.testng.TestNG testng.xml.This is how we can use TestNG listeners in Selenium.
Use of listeners in Selenium for multiple classes
Here’s an example of how to use TestNG listeners in Selenium for multiple classes by adding the <listener> tag in the testng.xml file:
- We create the listener class “MyListener” as previously.
- We create multiple test classes that we want to run, for example:
- We add the listener class to the testng.xml file by adding it to the listeners tag:
- You can also add the test classes to the testng.xml file inside the <classes> tag:
- We run the testng.xml file as usual, and the listener methods will be invoked at the appropriate time during the test execution for both classes.
In this example, we have added the listener class “MyListener” to the testng.xml file by adding it to the listeners tag. This will be applied to all classes that are added to the <classes> tag in the same file. Here we have added two classes TestClass1 and TestClass2 to the <classes> tag inside the <test> tag. So, during the execution of the tests, whenever a test will be started, passed, failed, or skipped from both classes, the overridden methods of the listener class will be invoked.
Conclusion
In this article, we discussed the use of Listeners in Selenium as a powerful tool to add extra functionality to the tests. Moreover, we went through two types of listeners in Selenium – WebDriver listeners and TestNG listeners, and how they can be implemented in the test scripts.
WebDriver listeners are used to listening for events that occur in the browser such as navigating to a new URL or interacting with an element on the page. On the other hand, TestNG listeners are used to listening for events that occur in the TestNG framework such as the start and finish of a test or the success or failure of a test.
We also went through the process of adding the listener to the test script and testng.xml files. Overall, Listeners are a useful tool for logging, debugging, and adding additional behavior to your tests in Selenium.
Frequently Asked Questions
Why do we use listeners?
In Selenium, listeners are used to tracking events that occur during the execution of a test script. There are two types of listeners: WebDriver listeners and TestNG listeners.
A WebDriver listener can be used to track the navigation events, such as when a new page is loaded or a page refresh occurs. It can also be used to track the actions performed on web elements, such as clicking a button or entering text into a text field. TestNG listeners, on the other hand, are used to track events related to the TestNG framework. A TestNG listener can be used to take a screenshot when a test fails or to log information about the test execution.
What is the use of @listener annotation in TestNG?
The @Listener annotation in TestNG is used to specify a class that contains methods that should be invoked before or after certain events occur during a test run. These methods are called listener methods, and they can be used to perform additional actions such as logging, reporting, or cleanup.
For example, the @BeforeSuite annotation can be used to specify a method that should be run before the entire test suite starts, and the @AfterTest annotation can be used to specify a method that should be run after each test method.
What is the difference between a listener and a filter?
A listener and a filter in TestNG are both used to add additional functionality to a test suite, but they are used for different purposes. A listener in TestNG is a class that contains methods that are invoked before or after certain events occur during a test run.
For example, a listener might contain a method that is invoked before a test suite starts, or after a test method finishes. Listeners can be used to perform additional actions such as logging, reporting, or cleanup. A filter in TestNG, on the other hand, is used to control which test methods are run. A filter can be used to include or exclude certain test methods based on their attributes, such as their name or the group they belong to.