When it comes to testing through automation on a web application, Selenium is probably one of the primary choices for a tester. The rich library of methods that can detect and perform an action on any web element makes us wonder whether giving Selenium a try should be worth the effort.
In addition, when the open-source flag is seen waving high on a cloud repository, we are sure about our next steps and which way we are going through. This is not only my analysis of test automation and open-source inclination, but a lot of surveys also point toward the same results:
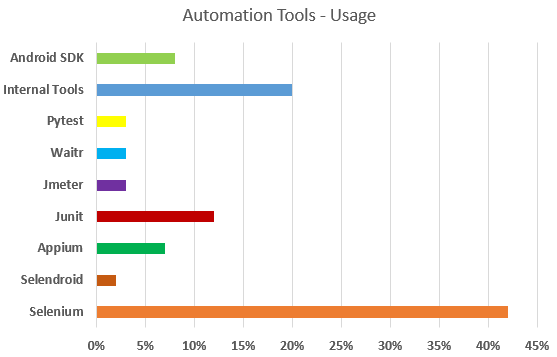
If we start discussing Selenium and its properties and everything it offers, we might end up covering up one book’s worth of material. Therefore, it is better to pick one of the most favorite and most used methods from the Selenium library and see how it helps us identify elements on a web page and take action on the results it returns. To demonstrate this and explore the process, we will use Selenium’s isDisplayed() method that tells us whether the element is displayed or not.
Table Of Contents
Isdisplayed() Method in Selenium
Unlike biologists and anthropologists, computer software developers and scientists name concepts extremely close to what they would achieve. So, navigate().to would navigate us to the specified URL, findElement() will find an element, and switchTo().window() will switch to another window.
Similarly, as you might have already guessed, isDisplayed() method in Selenium returns a boolean value telling us whether the element we are enquiring about is being displayed on the web page or not.
Do We Require Isdisplayed() Method in Selenium?
The first question that comes to mind is, do we need to dedicate one post to this method? Is this that important? I mean, not that I question the ability of Selenium Waits and the sense of relief it provides for the elements that load at different times, but can we rely on it entirely?
Let me rephrase the above sentence. If you have an element that does not load at the start, isn’t Selenium Waits enough to check if the element is available in the limited timeout we define in the method?
Selenium Waits let you wait for an element until it is visible (taking reference of a single use case out of many) on the web page. By its core nature, you have to wait for some time if you want to utilize this use case. I know you can implement waits with 0 value also, but calling internal functions and accessing multiple layers would increase the time of script execution.
As far as isDisplayed() is concerned, the method is instant and covers its own use case. Moreover, Selenium Waits do not return the boolean value as can be directly returned by the isDisplayed method in Selenium.
Know More: Page Loading in Selenium
How to Use Isdisplayed() Method in Selenium?
The isDisplayed method is a simple to learn and execute method. This section will demonstrate how to use it inside your scripts and check for an element on the web page. As a prerequisite, you need to be familiar with the concepts of Selenium and basic working around it, such as initializing a web driver and using the web driver for accessing the web-related elements.
The syntax for isDisplayed method in Selenium is as follows:
<webdriver instance>.<method to find element>(<property used>(<property symbol>)).isDisplayed();
I know it looks a bit intimidating due to many variables, especially for people just starting their Selenium journey. But no need to worry about that. It becomes much simpler when we boil this down to individual elements and understand the syntax more clearly.
<webdriver instance>
The webdriver instance is the instance of the webdriver you are using in Selenium. The webdriver instance is declared as:
WebDriver driver = new FirefoxDriver();
If you are using a Firefox driver or:
WebDriver driver = new ChromeDriver();
If you are using a Google Chrome driver.
<method to find element>
Here, we will ask Selenium to find an element. For this, we will use a method built into the Selenium library for finding an element such as findElement.
<property used>
After writing the method to find an element, we need to tell Selenium the property by which we are looking for that particular element. So, if I am finding an element with “div-header,” is it an ID? A class? Or a name property? Therefore, this is important to distinguish the two elements. In Selenium, you can use the following properties to find the element:
- Id
- ClassName
- CSS
- Name
- TagName
- Xpath
- LinkText
- PartialLinkText
After placing each of the values in the above attributes, our line of code for isDisplayed() method in Selenium would look as follows:
boolean isElementVisible = driver.findElement(By.id(“myHeaderDiv”)).isDisplayed();
Combining all the elements of code, we get the following snippet:
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class TestSigma {
protected WebDriver driver;
@Test
public void check_if_displayed()
{
driver = new ChromeDriver();
driver.navigate().to(“https://testsigma.com/blog/strings-in-javascript”);
boolean isElementVisible = driver.findElement(By.linkText(“Harish Rajora”)).isDisplayed();
System.out.println(“We found the element – ” + isElementVisible);
driver.close();
}
}
Here, I am using the linkText through findElement, which can find the text of a link. As expected, the result shown is true. Please note that we have used @Test annotations to work out this test. If you are unfamiliar with this, do read a detailed explanation on Parameterization in the TestNG post.
Isenabled() Method in Selenium
Since we are talking about finding elements on a web page, we may as well touch upon two more methods that are implemented with the same intentions.
The isEnabled() method in Selenium is used to find out whether an element is enabled on the web page (in the current browsing context) or not. The syntax of this method is as follows:
<webdriver instance>.<method to find element>(<property used>(<property symbol>)).isEnabled();
All the parameter attribute values remain the same as in isDisplayed method.
In the previous section, we verified whether the link text is displayed or not. Since it is a link, we can use the same code with the isEnabled() method in Selenium as follows:
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class TestSigma {
protected WebDriver driver;
@Test
public void check_if_enabled()
{
driver = new ChromeDriver();
driver.navigate().to(“https://testsigma.com/blog/strings-in-javascript”);
boolean isElementEnabled = driver.findElement(By.linkText(“Harish Rajora”)).isEnabled();
System.out.println(“We found the element – ” + isElementVisible);
driver.close();
}
}
This will also return the value true. This method is generally used for elements that are disabled depending on situations such as disabling the submit button unless the form is completely filled.
Isselected() Method in Selenium
The final element that we will consider in this post is the isSelected() method in Selenium. As its name suggests, the isSelected() method is used to find out whether an element is selected on the web page or not. With reference to the above two stated methods, this method resembles a lot in the syntax and the working. The syntax of the isSelected() method in Selenium is as follows:
<webdriver instance>.<method to find element>(<property used>(<property symbol>)).isSelected();
The meaning of various attributes defined in the syntax remains the same. The usage of isSelected is also extremely similar to the above:
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class TestSigma {
protected WebDriver driver;
@Test
public void check_if_selected()
{
driver = new ChromeDriver();
driver.navigate().to(“https://testsigma.com/blog/strings-in-javascript”);
boolean isElementSelected = driver.findElement(By.cssSelector(“input[type=’checkbox’]:indian-national”)).isSelected();
System.out.println(“We found the element – ” + isElementVisible);
driver.close();
}
}
Since the element differs from the one used in isEnabled() method, we have used cssSelector instead. If there are any doubts about the program execution, do let us know in the comment section, and we will be happy to clarify them.
Also, please note that the primary use case of the isSelected method in Selenium is around the elements that can be selected or are “checkable.” For example, this method will be most suitable to find out if a radio button is checked or a checkbox is checked or not, etc.
Conclusion
Selenium is inarguably one of the best platforms for scripted testing in the market. Even though credit goes out to its open-source nature and the large community that supports us in every hurdle, a lot of other things contribute to its success too.
For instance, developers have always maintained a habit of listening to testers and implementing the most demanding features as soon as possible. They have also kept the concepts and the working of each method considerably simple compared to other frameworks. In this post, we tried to pick out three such things from the large pool and see how they can help us in testing web applications faster and more efficiently.
The isDisplayed() method in Selenium is used to test whether the referenced element is displayed on the web page or not. It returns a boolean value and can be used with any locator found in Selenium. Unlike Selenium Waits, the result is instant and requires fewer calls within.
In addition, we discussed two more similar functions that are used exactly the same way as isDisplayed method with specific use cases. For instance, if you are using isEnabled() method in Selenium, its expertise falls within links, buttons, etc., that can be enabled.
Similarly, isSelected() method works best where there are checkable elements on the web page. Apart from these three methods, you can use getTagName, getRect, etc. methods to get element-specific information. However, their motive deviates us from the essence of this post, and so we would like to wrap things up here.
Please let us know in the comment section if you have any suggestions, queries, or comments. Thank you for giving this post your valuable time.
Suggested Readings
Selenium SendKeys: A Detailed Usage Guide
Selenium Get Current URL using Java: Tutorial