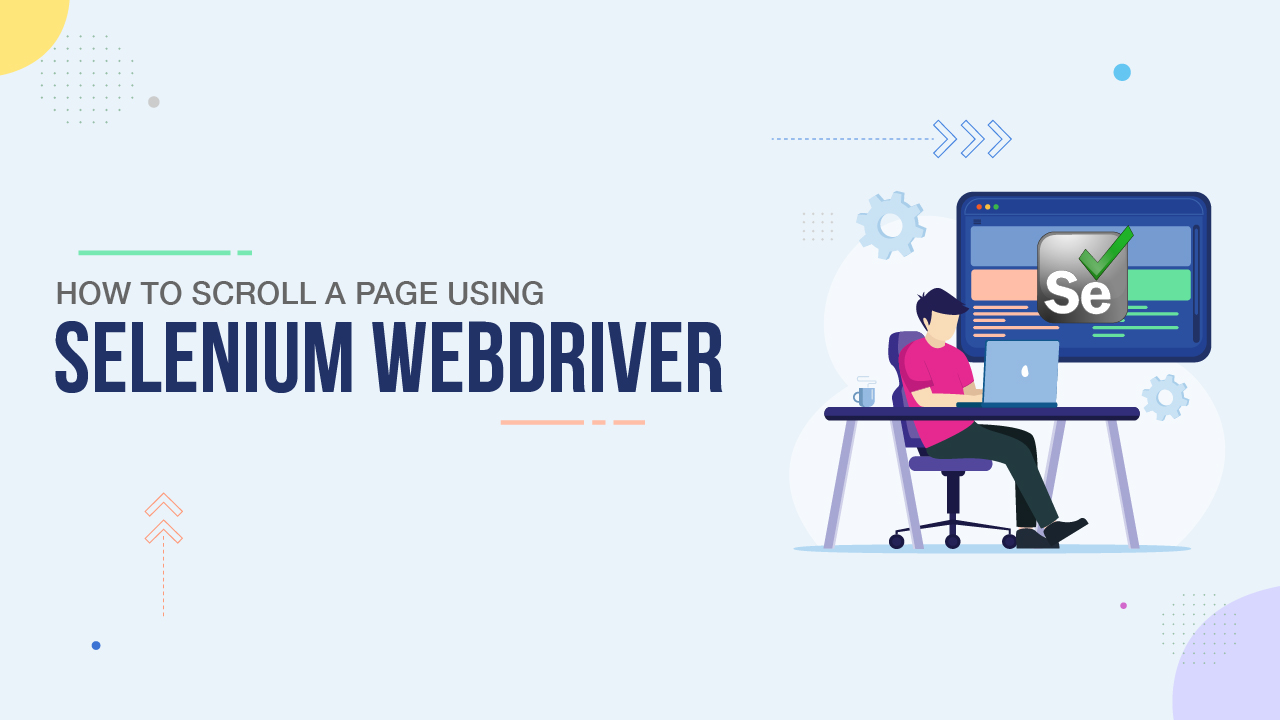
Scrolling is one of the most important features of any application. You will not find any website which does not have scrolling capability. It’s almost impossible to display all the content in a single frame, users need to scroll down or up to get all the content of the application. This article will help you to understand horizontal and vertical scroll operations with Selenium WebDriver.
Table Of Contents
- 1 What is the Purpose of Scrolling in Selenium WebDriver?
- 2 How to Scroll Down in Selenium?
- 3 How do I Scroll based on the Visibility of the Web element on the page in Selenium?
- 4 How does Selenium Scroll a Webpage both Horizontally and Vertically?
- 5 How do you Scroll a Webpage with Infinite Scrolling using Selenium?
- 6 How do you Scroll to the Top of the Page in Selenium?
- 7 How do you Scroll Down a Page by Specified Pixels in Selenium?
- 8 Best Practices for Scrolling in Selenium
- 9 Scroll A Page With Low/No-code: Testsigma
- 10 Conclusion
- 11 Frequently Asked Question
What is the Purpose of Scrolling in Selenium Webdriver?
Largely, scrolling in Selenium WebDriver is used to automate user interaction with web elements not immediately visible within the viewport. Since many web pages use lazy loading, infinite scrolling, and elements being with a scrollable container, automating scrolling is necessary for running tests on web pages.
The purpose of scrolling in Selenium WebDriver includes the following:
- Interacting with off-screen elements: Certain elements are situated outside the immediate viewport, and scrolling makes these elements appear so they can be clicked.
- Managing lazy loading: Certain websites will lose content dynamically, as the page is scrolled. In Selenium tests, scrolling works to ensure that these elements are available for interactions.
- Dealing with infinite scrolling: Certain web pages will continuously load content as the user scrolls. In this case, automated scrolling is needed to load new elements dynamically.
- Capturing screenshots: If a test requires full-page screenshots, automated scrolling is needed to capture all parts of the webpage.
- Checking UI responsiveness: Automating scrolling enables the test engine to navigate to different parts of a page — this is needed to verify layout changes, floating elements, and sticky components.
Starting your journey with Selenium WebDriver? Check the article on Selenium WebDriver.
In Selenium, scrolling can be handled by using JavaScriptexecutor. JavaScriptexecutor is an interface that is used to execute JavaScript through selenium webdriver. The JavaScript Executor is a powerful feature of Selenium WebDriver that allows you to execute JavaScript code directly in the browser.
JavaScriptexecutor provides two methods:
- ExecuteScript
- ExecutrAsyncScript
Syntax:
JavaScriptexecutor js = (JavascriptExecutor) driver; js.executeScript(“window.scrollBy(0,350)”, “”);ScrollBy is a JavaScriptexecutor method that accepts two parameters x and y, which defines horizontal and vertical axes.
Now let’s discuss the Scroll operation in different scenarios:
- How do I scroll down to the page’s bottom using Selenium?
- How do I scroll based on the visibility of the Web element on the page in Selenium?
- How does Selenium scroll a webpage both horizontally and vertically?
- How do you scroll a webpage with infinite scrolling using Selenium?
- How do you Scroll to the top of the page in Selenium?
- How do you scroll down in a page by specified pixels in Selenium?
How to Scroll down in Selenium?
In Selenium, you can use the JavaScript Executor to scroll down to the bottom of a web page. You can use the “scrollTo” method to scroll to the bottom of the page. The following code snippet shows an example of how to scroll down to the bottom of a web page using Selenium in Java:
<javascriptexecutor js=”(JavascriptExecutor)” driver;=”” js.executescript(“window.scrollto(0,=”” document.body.scrollheight)”);In the above example, the JavaScript Executor is first cast to a JavascriptExecutor object and then the “executeScript” method is used to execute a JavaScript code. The JavaScript code passed to the “executeScript” method is “window.scrollTo(0, document.body.scrollHeight)”, which scrolls the page to the bottom.
Code snippet:
import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ScrollDown { public static void main(String[] args) { //specify the location of the driver System.setProperty(“webdriver.chrome.driver”,”ChromeDriver_path”); //Initialising the driver WebDriver driver = new ChromeDriver(); //launch the application driver.get(“https://opensource-demo.orangehrmlive.com/web/index.php/auth/loginweb/index.php/auth/login”); //maximize the browser driver.manage().window().maximize(); //It will wait for maximum of 10sec for each object driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); //Identify username field using xpath as locator WebElement username = driver.findElement(By.xpath(“//input[@placeholder=’Username’]”)); // Identify password field using xpath as locator WebElement password = driver.findElement(By.xpath(“//input[@placeholder=’Password’] “)); //Enter the value of the username username.sendKeys(“Admin”); //Enter the value of the password password.sendKeys(“admin123”); //identify the locator of the login button and click on Login btn driver.findElement(By.id(“btnLogin”)).click(); JavascriptExecutor js = (JavascriptExecutor) driver; //It will help you to get the height of the page and helps to scroll to the bottom of the page js.executeScript(“window.scrollTo(0, document.body.scrollHeight)”); driver.close(); } }Above code snippet will help you to scroll your page to the bottom of the page.
How Do I Scroll Based on the Visibility of the Web Element on the Page in Selenium?
Before you perform scroll operations in selenium, it is necessary that the corresponding web element is present under DOM.
ScrollIntoView() method will help you to scroll to the desired element.
The scrollIntoView() method is a JavaScript method that can be used to scroll an HTML element into the viewport. When called on an element, it will scroll the element’s parent container so that the element becomes visible in the viewport.
JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript(“arguments[0].scrollIntoView();”, webElement);Code snippet:
package demo; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ScrollIntoTheView { public static void main(String[] args) { //specify the location of the driver System.setProperty(“webdriver.chrome.driver”,”ChromeDriver_path”); //Initialising the driver WebDriver driver = new ChromeDriver(); //launch the website driver.get(“https://testsigma.com/”); //maximize the window to full screen driver.manage().window().maximize(); //It will wait for maximum of 10sec for each object driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); JavascriptExecutor js = (JavascriptExecutor) driver; //Identify the xpath of the object. WebElement element = driver.findElement(By.xpath(“//span[text()=’Sign up for free’]”)); //scrollIntoView will help us to scroll to the Sign-up button js.executeScript(“arguments[0].scrollIntoView();”, element); driver.close(); } }How Does Selenium Scroll a Webpage Both Horizontally and Vertically?
In Selenium, you can use the JavaScript Executor to scroll a web page both horizontally and vertically. However, the JavaScript Executor does not have the method to scroll a web page both horizontally and vertically. It has the method “scrollBy” and “scrollTo” which can scroll the page horizontally or vertically.
In order to scroll the page both horizontally and vertically, you need to use the “scrollBy” method twice in a row, once for horizontal scrolling and once for vertical scrolling. Here is an example of how to use the “scrollBy” method to scroll a web page both horizontally and vertically in Selenium:
JavaScriptexecutor js = (JavascriptExecutor) driver; js.executeScript(“window.scrollBy(3000,0)”); //Horizontal scroll js.executeScript(“window.scrollBy(0,3000)”); //Vertical ScrollCode snippet:
For Horizontal Scroll
package demo; import java.util.concurrent.TimeUnit; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class HorizontalScroll { public static void main(String[] args) { //specify the location of the driver System.setProperty(“webdriver.chrome.driver”,”ChromeDriver_path”); //Initialising the driver WebDriver driver = new ChromeDriver(); //launch the website driver.get(“https://www.album.alexflueras.ro/index.php”); //maximize the window to full screen driver.manage().window().maximize(); //It will wait for maximum of 10sec for each object driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript(“window.scrollBy(5000,40)”); driver.close(); } }
For Vertical Scroll
package demo; import java.util.concurrent.TimeUnit; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class VerticalScroll { public static void main(String[] args) { //specify the location of the driver System.setProperty(“webdriver.chrome.driver”,”ChromeDriver_path”); //Initialising the driver WebDriver driver = new ChromeDriver(); //launch the website driver.get(“https://www.album.alexflueras.ro/index.php”); //maximize the window to full screen driver.manage().window().maximize(); //It will wait for maximum of 10sec for each object driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript(“window.scrollBy(40,3000)”); //vertical scroll driver.close(); } }How Do You Scroll a Webpage with Infinite Scrolling Using Selenium?
In today’s world, most applications implement infinite scrolling. Since users do not have to do the time-consuming operation of pressing the “Previous” and “Next” buttons, pages with endless scrolling may see improved engagement.
You can visit https://the-internet.herokuapp.com/infinite_scroll to look at infinite scrolling.
Code snippet:
package demo; import java.util.concurrent.TimeUnit; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class InfiniteScrolling { public static void main(String[] args) { //specify the location of the driver System.setProperty(“webdriver.chrome.driver”, “ChromeDriver_path”); //Initialising the driver WebDriver driver = new ChromeDriver(); //launch the website driver.get(“https://www.album.alexflueras.ro/index.php”); //maximize the window to full screen driver.manage().window().maximize(); //It will wait for maximum of 10sec for each object driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); JavascriptExecutor js = (JavascriptExecutor) driver; long intialLength = (long) js.executeScript(“return document.body.scrollHeight”); while(true){ js.executeScript(“window.scrollTo(0,document.body.scrollHeight)”); try { Thread.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); } long currentLength = (long) js.executeScript(“return document.body.scrollHeight”); if(intialLength == currentLength) { break; } intialLength = currentLength; } } }How Do You Scroll to the Top of the Page in Selenium?
In the above article, we have seen scrolling down or scrolling horizontally but scrolling to the top of the page is one of the widely used operations on pages using the JavascriptExecutor interface.
To demonstrate this, we first need to scroll down to the page using selenium and then scroll up to the page.
There are various ways to scroll to the top of the page.
Method 1:
package demo; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ScrollUp { public static void main(String[] args) { //specify the location of the driver System.setProperty(“webdriver.chrome.driver”,”ChromeDriver_path”); //Initialising the driver WebDriver driver = new ChromeDriver(); //launch the website driver.get(“https://www.album.alexflueras.ro/index.php”); //maximize the window to full screen driver.manage().window().maximize(); //It will wait for maximum of 10sec for each object driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); WebElement username = driver.findElement(By.xpath(“//input[@placeholder=’Username’]”)); WebElement password = driver.findElement(By.xpath(“//input[@placeholder=’Password’] “)); username.sendKeys(“Admin”); password.sendKeys(“admin123”); WebElement loginBtn = driver.findElement(By.id(“btnLogin”)); loginBtn.click(); JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript(“window.scrollTo(0, document.body.scrollHeight)”); try { Thread.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); } js.executeScript(“window.scrollTo(document.body.scrollHeight,0)”); driver.close(); } }How Do You Scroll down a Page by Specified Pixels in Selenium?
With the help of JavaScriptExecutor, you can scroll to a particular point by specifying the exact pixel or coordinates of the object. This can be achieved by using the ‘ScrollBy’ function.in JavaScript.
Code Snippet:
package demo; import java.util.concurrent.TimeUnit; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class ScollByPixel { public static void main(String[] args) { //specify the location of the driver System.setProperty(“webdriver.chrome.driver”,”ChromeDriver_path”); //Initialising the driver WebDriver driver = new ChromeDriver(); //launch the website driver.get(“https://www.album.alexflueras.ro/index.php”); //maximize the window to full screen driver.manage().window().maximize(); //It will wait for maximum of 10sec for each object driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); JavascriptExecutor js = (JavascriptExecutor) driver; //specify the number of pixels the page must be scrolled js.executeScript(“window.scrollBy(0,3000)”); driver.close(); } }Best Practices for Scrolling in Selenium
Efficient scrolling in Selenium is needed to create reliable automation scripts that can handle dynamic content, infinite scrolling, and hidden elements. Some best practices in this regard are:
- Scroll directly to a single element instead of scrolling by a fixed number of pixels.
- Use JavaScript Executor to handle scrolling only if WebDriver methods fail.
- Scrolling should be automated in small increments.
- Verify if any new elements have appeared after each scroll.
- Don’t use hardcoded waits. Instead of Thread.sleep, use Explicit Waits to instruct the test script to wait dynamically for loading elements.
- Use Actions Class to automate scrolling via keyboard or mouse.
- Check that scrolling behavior remains consistent across browsers — Chrome, Firefox, and Edge handle scrolling differently from each other.
- Test scrolling and elements in different viewport sizes. Use Selenium’s setWindowSize to test responsive designs.
- Minimize unnecessary scrolling by using it only to speed up test execution.
- Instruct the script to check if an element is visible before starting to scroll.
Learn more about Selenium Automated Testing
Scroll a Page with Low/no-code: Testsigma
Alternatively, there is a quick and efficient way to automate this test scenario. You can leverage Testsigma’s low-code techniques and create robust test steps to automate scrolling in less than a minute.
Let’s take an example: Consider a user who wants to look up available flights from A to B, where they scroll through various relevant results to find the ultimate one. This can be done in a few steps with less time and effort, yet efficiently using codeless testing features supported by Testsigma, like NLPs, Record & Playback, and Data-driven testing.
Look at the screenshot below, where scrolling is automated using a Scroll NLP.
Check out the comprehensive comparison of Selenium vs Testsigma and how they impact the test automation for your business in terms of speed, efficiency, cost, test ROI, and more.
Conclusion
In the above article, we have seen different ways of scrolling on a web page using Selenium with Java. One of the frequently performed tasks when it comes to Selenium automation testing is automating the page scroll action utilizing the JavaScriptExecutor interface. We have seen sample code for scrolling down, scrolling up, scrolling horizontally and vertically, etc. Do share your thoughts on how you have implemented scrolling a web page in your scripts.
Do share this blog with your friends and colleagues.
Happy testing 😊
Frequently Asked Question
How Do I Scroll down Using Sendkeys in Selenium?
In Selenium, you can use the sendKeys() method to simulate key presses, including the “Page Down” key. To scroll down using sendKeys(), you can use the following code:
Actions action = new Actions(driver); action.sendKeys(Keys.PAGE_DOWN).build().perform();This will simulate a “Page Down” key press on the element, which will scroll the element down.
How Do I Scroll down a Page Using Java by Specified Pixels in Selenium?
In Selenium using Java, you can use the JavascriptExecutor class to execute JavaScript code that can scroll the scroll bar on a page. Here is an example of how you can scroll the scroll bar down by a certain number of pixels:
JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript(“window.scrollBy(0,1000)”);This will scroll down the web page by 1000 pixels. You can adjust the number of pixels to scroll by as needed.
Can I Automate Infinite Scrolling in Selenium Webdriver?
Yes, you can automate infinite scrolling in Selenium WebDriver.
How Do I Handle Dynamic Content While Scrolling in Selenium?
Handling dynamic content while scrolling can be a bit complex. But it is doable; follow the steps to handle dynamic content.
- Load the page and initialize.
- Set the rule for detecting dynamic content
- Wait for the new content to load and compare it with the previous version.