In this article, let’s discuss the differences between Cypress vs React Testing Library. We will understand their use cases and limitations in detail, the major differences between them, and also discuss a better test automation alternative to Cypress and React testing library.
Table Of Contents
- 1 Cypress vs React Testing Library – Overview
- 2 Cypress vs React Testing Library: What are the Differences?
- 3 Similarities between Cypress and React Testing Library
- 4 Cypress vs React Testing Library: When to Use Which?
- 5 Cypress vs React Testing Library: Which is Better?
- 6 Testsigma vs Cypress vs React Testing Library
- 7 Conclusion
- 8 Frequently Asked Questions
Cypress VS React Testing Library – Overview
Choosing the right test automation tool depends on your testing goals. Both Cypress and React Testing Library have their own set of features and use cases. Let’s have a look at what each of them brings to the table.
Cypress
Cypress is an open-source end-to-end (E2E) testing framework designed for modern web applications. It provides a visual interface for writing and debugging tests, mimicking real user interactions with the browser. Cypress runs tests within a real browser environment, allowing for testing complex workflows, user journeys, and integration with external APIs.
You can also check out the top 10 Cypress test automation alternatives.
Features of Cypress
- End-to-End Testing: Cypress excels at simulating user interactions and testing the entire application from a user’s perspective.
- Visual Testing: It allows for visual regression testing to ensure UI remains consistent across changes.
- Time Travel Debugging: Cypress enables debugging tests by stepping through them and inspecting the application state at any point.
- Network Stubbing: Network requests and responses can be controlled and mocked for testing various scenarios.
- Cross-Browser Testing: Cypress supports testing across different browsers with minimal configuration.
Check here – Playwright vs Cypress
React Testing Library
React Testing Library is a lightweight testing library specifically designed for testing React components in isolation. It encourages writing tests that mimic user interactions and focuses on component behavior rather than implementation details. This isolation helps ensure components are reusable and maintainable.
Features of React Testing Library
- Component-Focused Testing: React Testing Library promotes testing components in isolation, ensuring they function as intended regardless of integration with other components. Check here – Component Testing
- Focus on User Interactions: It encourages writing tests that simulate real user interactions with components, leading to more user-centric tests.
- Accessibility Testing: The library provides utilities for testing component accessibility according to best practices.
- Minimal DOM Assertions: It avoids making assumptions about the component’s internal structure, promoting more maintainable tests.
- Easy Setup: React Testing Library integrates well with existing React testing frameworks like Jest.
Check here – Cypress vs Selenium
Cypress VS React Testing Library: What Are the Differences?
Let’s have a look at the key differences between Cypress and React Testing Library in terms of pros, cons, scope, and other features.
Pros – Cypress VS React Testing Library
Pros | Cypress | React Testing Library |
Comprehensiveness | Offers a wide range of features for E2E testing | Focuses on isolated component testing, promoting reusability |
Debugging | Enables time-travel debugging and state inspection | Not directly supported |
Visual Testing | Supports visual regression testing | Not directly supported |
Network Control | Allows mocking and controlling network requests | Limited support for network request mocking |
Cross-Browser Testing | Supports testing across different browsers | Primarily focused on testing in a single browser (though workarounds exist) |
Ease of Use | Relatively easy setup | Straightforward setup with existing frameworks like Jest |
Learning Curve | A steeper learning curve due to a broader feature set | Generally considered easier to learn due to focused functionality |
Cons of Cypress VS React Testing Library
Cons | Cypress | React Testing Library |
Test Speed | Tests can be slower to execute due to complexity | Generally faster test execution due to isolated testing |
Overkill for Simple Apps | Extensive features might be unnecessary for basic apps | Limited for complex interactions between components |
Accessibility Testing | Limited built-in support, but can be extended | Can be used with utilities to test component accessibility |
Scope and Purpose – React Testing Library VS Cypress
Feature | Cypress | React Testing Library |
Testing Approach | Simulates real user journeys across the application | Focuses on verifying individual component functionality |
DOM Interaction | Extensive DOM manipulation capabilities (modify elements, trigger events) | Provides utilities to query and interact with specific component parts |
Match Assertions | Built-in matchers for DOM element states (visibility, text content) | Uses libraries like Jest for assertions on rendered components and interactions |
Network Stubbing | Controls and mocks network requests and responses | Limited support for mocking network requests (workarounds with external libs) |
Integration Testing | Well-suited for testing integration between components and external APIs | Limited capability for integration testing by default |
Community & Support | Large and active community with extensive resources | Growing community with good support, but smaller than Cypress |
Examples of Cypress and React Testing Library
Imagine a content management system (CMS) for a blog where users can create new blog posts with titles, content, and featured images. Let’s explore how Cypress and React Testing Library can be used to test different aspects of this functionality:
Scenario 1: Creating a New Blog Post with Image Upload (Cypress)
This scenario tests the user journey of creating a new blog post, including filling out the form, uploading an image, and saving the post. Cypress simulates user interactions and verifies successful post creation.
describe(‘Blog Post Creation’, () => { it(‘allows creating a new post with image upload’, () => { cy.visit(‘/new-post’); cy.get(‘#title’).type(‘My Awesome Blog Post’); cy.get(‘#content’).type(‘This is some great content for the blog post.’); // Simulate image upload using custom Cypress command cy.get(‘#featured-image’).attachFile(‘images/test-image.jpg’); cy.get(‘button[type=”submit”]’).click(); cy.get(‘.success-message’).should(‘be.visible’).and(‘contain’, ‘Post Created Successfully!’); cy.get(‘.post-title’).should(‘contain’, ‘My Awesome Blog Post’); // Additional assertions to verify image is displayed in the post preview }); }); // Custom Cypress command for attaching file (example) Cypress.Commands.add(‘attachFile’, (selector, filePath) => { cy.get(selector).selectFile({ force: true }, filePath); }); |
Explanation:
- We visit the new post creation page.
- We interact with form fields using Cypress commands to fill in the title and content.
- We define a custom Cypress command attachFile to simulate selecting and uploading an image from the filesystem.
- We submit the form and verify success messages and post details.
- We can add additional assertions to verify the uploaded image is displayed within the post preview.
Scenario 2: Validating Title and Content Input (react Testing Library)
This scenario focuses on testing the behavior of the title and content input fields within the blog post creation form using the React Testing Library. We’ll isolate the form component for focused testing.
import { render, screen } from ‘@testing-library/react’; import userEvent from ‘@testing-library/user-event’; import PostForm from ‘./PostForm’; // Assuming PostForm component test(‘validates required title field’, () => { render(<PostForm onSubmit={jest.fn()} />); userEvent.type(screen.getByLabelText(‘Content’), ‘This is some content.’); userEvent.click(screen.getByText(‘Submit’)); expect(screen.getByText(‘Error: Title is required’)).toBeInTheDocument(); }); test(‘validates content length’, () => { render(<PostForm onSubmit={jest.fn()} />); userEvent.type(screen.getByLabelText(‘Title’), ‘A Short Title’); userEvent.type(screen.getByLabelText(‘Content’), ‘Short content’); userEvent.click(screen.getByText(‘Submit’)); expect(screen.getByText(‘Error: Content must be at least 50 characters long’)).toBeInTheDocument(); }); |
Explanation:
- We render the PostForm component in isolation.
- In the first test, we simulate user interaction by typing content but leaving the title blank. We then submit the form and verify an error message for the missing title.
- In the second test, we simulate typing a short title and content that doesn’t meet a minimum character requirement. Submitting the form should display a validation error message.
Cypress is ideal for testing the entire user experience of creating a new blog post with image upload, ensuring smooth interaction with the form, image upload functionality, and successful post creation.
React Testing Library excels at isolating the PostForm component and verifying its behavior regarding required fields and content length validation, promoting robust form functionality.
Check here – Cypress vs Jest
Similarities between Cypress and React Testing Library
While Cypress and React Testing Library serve different testing purposes, they share some underlying principles:
- Focus on User Interactions: Both libraries encourage writing tests that mimic how users interact with the application. This ensures tests capture real-world behavior and potential issues users might encounter.
- JavaScript-Based: Both tools are written in JavaScript, allowing them to seamlessly integrate with your React application codebase. This simplifies test development and maintenance as developers can leverage their existing JavaScript knowledge.
- DOM Manipulation: Both libraries provide methods for interacting with the Document Object Model (DOM). Cypress offers a more comprehensive set of commands for interacting with various DOM elements, while React Testing Library provides utilities for finding specific elements within a component’s rendered output.
- Asynchronous Testing Support: Both Cypress and React Testing Library offer mechanisms for writing asynchronous tests, allowing you to verify behavior that relies on these operations. For instance, Cypress provides commands like “cy.wait()” and promises to handle asynchronous actions, while React Testing Library can be used with libraries like “@testing-library/user-event” to simulate user interactions and wait for their effects.
- Matchers: Both tools allow for asserting expected outcomes from your tests. Cypress provides built-in matchers like “.should(‘be.visible’)” or “.should(‘have.text’, ‘expected text’)” to verify DOM element states. Similarly, React Testing Library offers utilities like “expect(element).toBeInTheDocument()” or “expect(element).toHaveTextContent(‘expected text’)” to make assertions on rendered components.
- Custom Commands/Components: Both Cypress and React Testing Library allow you to extend their functionality by creating custom commands or components.
Check here – Cypress vs Puppeteer
Cypress VS React Testing Library: When to Use Which?
Choosing between Cypress and React Testing Library depends on the scope and goals of your tests. Here’s a breakdown of their ideal use cases:
Cypress Use Cases
- End-to-End Testing: You need to simulate real user journeys across your application, involving multiple components and interactions.
- Visual Testing: Ensuring consistent UI across changes is crucial. Cypress allows for visual regression testing to catch UI inconsistencies.
- Integration Testing: Testing interactions between your application and external APIs or services is a primary focus.
- Complex Workflows: Your application involves intricate workflows that require testing various user interactions and outcomes.
React Testing Library Use Cases
- Component-Level Testing: You want to isolate and test individual React components to ensure they render and function correctly on their own.
- Reusable Components: Focusing on testing reusable components in isolation helps maintain their functionality and avoid regressions.
- User Interactions: Simulating user interactions with specific components and verifying their expected behavior is a priority.
- Accessibility Testing: While not its core purpose, React Testing Library can be used with utilities to test the accessibility of components.
In essence, Cypress is ideal for testing the entire user experience, while React Testing Library focuses on the building blocks (components) that make up that experience.
Cypress VS React Testing Library: Which is Better?
There’s no single “better” choice between Cypress and React Testing Library. They serve different purposes and excel in distinct areas of testing. The ideal tool depends on your specific testing needs and project context. Here’s a breakdown to help you decide:
Choose Cypress if:
- You prioritize end-to-end testing that simulates real user journeys across your application.
- Visual regression testing is crucial to ensure consistent UI across changes.
- Your application relies heavily on integration with external APIs or services.
- You need to test complex workflows involving various user interactions and outcomes.
Choose React Testing Library if:
- Your focus is on component-level testing to ensure individual React components render and function correctly in isolation.
- You want to promote reusable components by verifying their behavior independently.
- Testing user interactions with specific components is a primary concern.
- You value a faster test execution speed and a more concise testing approach.
Cypress might have a steeper learning curve due to its broader feature set. React Testing Library is generally considered easier to learn for its focused functionality.
In many cases, you might find it beneficial to use both tools together. Cypress provides a solid foundation for end-to-end testing, while React Testing Library complements it by ensuring the building blocks (components) function as intended. This combined approach leads to more comprehensive and robust test coverage.
Testsigma VS Cypress VS React Testing Library
While Cypress and React Testing Library are powerful tools, they have limitations that might necessitate exploring alternative solutions. Cypress excels at user journey simulations but might be overkill for unit testing isolated components. Moreover, comprehensive features can lead to slower test execution times, impacting development speed.
On the other hand, React Testing Library is excellent for React components, but might not be ideal for testing non-React components or vanilla JavaScript functionalities. While workarounds exist, React Testing Library has limited built-in support for testing interactions between separate components.
Why Consider Testsigma As an Alternative?
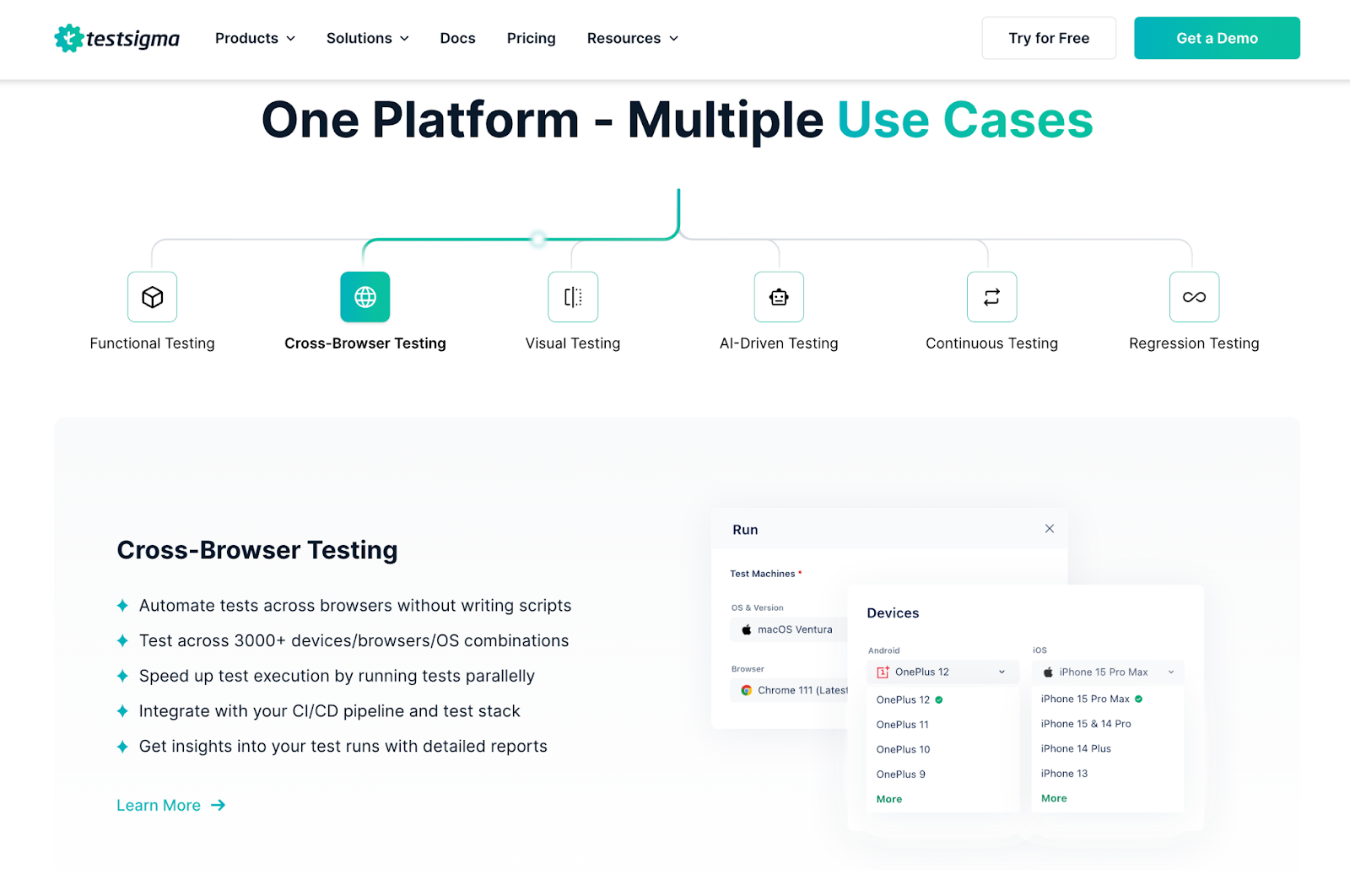
Testsigma offers a comprehensive testing solution that addresses some of the limitations of Cypress and React Testing Library. Testsigma is a cloud-based test automation platform that supports a wide range of testing needs. It allows you to write automated tests for web, mobile, and API applications using a visual interface and simple English natural language. Here’s how easy it is to create a test case using Testsigma.
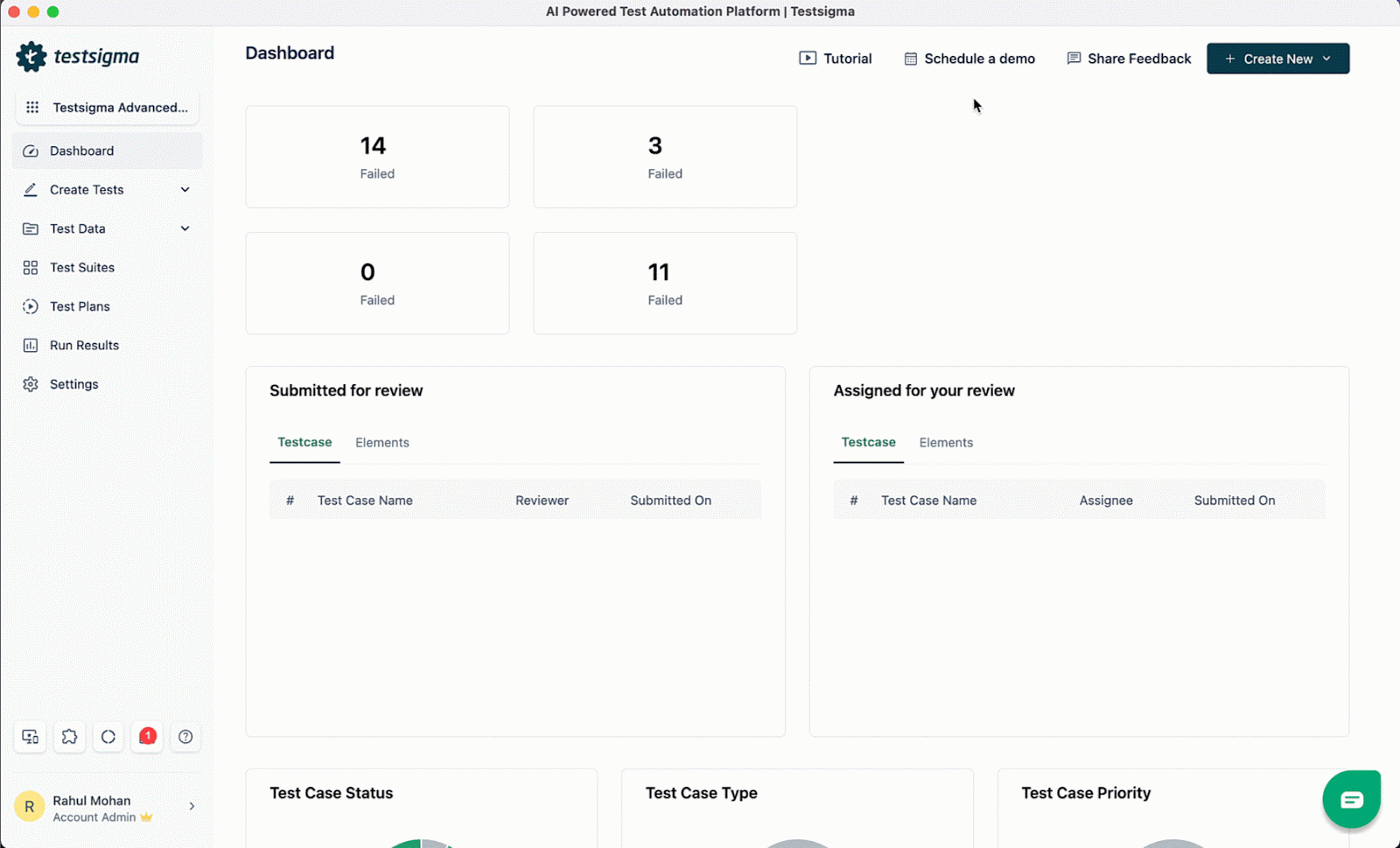
Highlighting Features of Testsigma
- Unified Platform: Supports testing web, mobile, and API applications from a single platform.
- Visual & Script-Based Testing: Offers both visual recording and scripting capabilities for test creation.
- AI-Powered Maintenance: Utilizes AI to automatically generate healing scripts when application elements change.
- Cross-Browser Testing: Enables testing across different browsers and devices on a cloud grid.
- Parallel Execution: Allows running tests in parallel for faster test execution times.
- Data-Driven Testing: Supports data-driven testing using external data sources (CSV, Excel, databases).
- Integrations: Integrates with popular CI/CD tools and project management platforms.
- Detailed Reporting: Provides comprehensive test reports with screenshots, logs, and analytics.
- Scalability: Cloud-based platform scales to accommodate growing testing needs.
- User-Friendly Interface: Offers an intuitive interface for both technical and non-technical users.
Conclusion
In conclusion, choosing the right test automation tool depends on your specific testing goals and project context. Cypress excels in end-to-end testing, simulating user journeys and interactions across your application. React Testing Library shines in component-level testing, ensuring individual components function correctly in isolation.
While both tools are valuable, they have limitations. Testsigma emerges as a potential alternative, offering a unified platform for web, mobile, and API testing with features like visual recording, AI-powered maintenance, and parallel execution. Ultimately, the best approach might involve combining tools based on your testing needs. Consider factors like learning curve, project requirements, and team skillset when selecting the most suitable solution for your development workflow.
Frequently Asked Questions
Is Cypress the Way to Go with React Component Testing?
Cypress is not the ideal choice for React component testing. While it allows interaction with components, its focus lies in end-to-end testing and user journey simulation. For isolated component testing and ensuring their functionality, React Testing Library with its user-centric approach and utilities for rendering and interacting with components in isolation is a better fit.
How to Do Integration Test with Cypress OR React Testing Library?
Cypress is well-suited for integration testing. It offers features like mocking network requests and controlling application state, allowing you to test how components interact with external APIs or services. React Testing Library, on the other hand, has limited built-in support for integration testing. However, you can combine it with mocking libraries or frameworks like Jest to simulate external interactions for basic integration testing needs.
Can We Migrate Test DATA From Cypress OR React Testing Library to Testsigma?
Testsigma supports data-driven testing using external data sources. While Cypress and React Testing Library don’t directly export test data, you can potentially migrate the data itself (CSV, Excel files) to be used within Testsigma. The test scripts themselves might require some adjustments to leverage Testsigma’s data-driven testing functionalities effectively.