Start automating your tests 10X Faster in Simple English with Testsigma
Try for freeIt is high time we put out an edition of the GraphQL tutorial for our QA teams. There is a lot of material and more confusion on how to create GraphQL APIs on the internet. As if the idea of REST was easy for teams to understand – the definition of REST has always stopped just at the abbreviation, Representational State Transfer. Developers and Testers alike don’t really understand the concept. We know how to create REST APIs and how to test them and that works for us.
And while we were already fighting to understand REST APIs, we are told that there is a new kid on the block. Many companies including Coursera have moved onto GraphQL. You have to know how to test this new API that twitter and Starbucks are using to pull data.
So here we go, a simplified version of the GraphQL story for the testing community.
We will follow a simple sequence of steps to first understand about APIs and REST APIs. Then we travel to the realms of GraphQL and understand how they are different. Finally we create a master plan to test them. Well, aren’t we here to do just that.
Table Of Contents
- 1 API ? What is that?
- 2 Building a REST API in 5 Minutes
- 3 A Simple GraphQL Application
- 4 Testing a GraphQL API
- 5 What is GraphQL Testing?
- 6 What Needs to be Tested in the GraphQL Test?
- 7 The Importance of Testing GraphQL APIs
- 8 Components to Test in GraphQL
- 9 Types of GraphQL API testing
- 10 Testing GraphQL Best Practices
- 11 GraphQL Testing Tools
- 12 Automate Your GraphQL Tests with Testsigma
- 13 What is GraphQL Compared to REST?
- 14 Frequently Asked Questions
API ? What is that?
API is the acronym for Application Programming Interface, which is a software intermediary that allows two applications to talk to each other.
Each time you use an app like Facebook, send an instant message, or check the weather on your phone, you’re using an API.
Let us take the example of a restaurant :
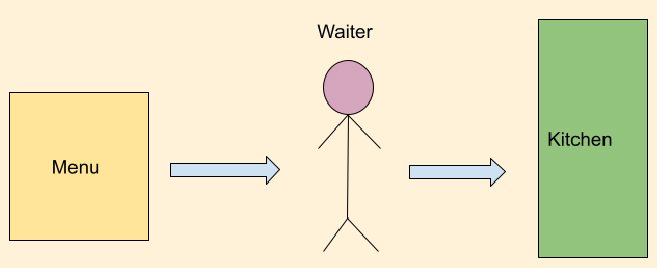
Stick man representing a waiter. Boxes representing other elements.
Assume you are in a restaurant and you have the menu in your hands.
For the restaurant kitchen to understand what you want to eat/order, you need to communicate this to Kitchen via the menu.
Here we have two different systems – You & the restaurant kitchen.
The waiter is the API, takes orders from you, passes it to the kitchen, gets the food to your table.
So now, we know briefly about what an API is. It helps two systems communicate.
Now we will try and understand what a REST API is.
Building a REST API in 5 Minutes
To understand the life of a “Waiter”, you have to understand the restaurant ecosystem.
Let us build a tiny little REST API using nodejs and express.js
You don’t know nodejs and expressjs ? Don’t worry. The objective of this simple project is to simply show you the API that we create using these frameworks.
Just follow these simple steps.
What this essentially means is, we will be deploying our API on express js server.
Step 1
Download and install NodeJS.
https://nodejs.org/en/download/
Step 2
Check if node and node package manager are installed
Open your command prompt or terminal and type the following commands one by one
npm -v
node -v
If these statements give you valid results, you have node and node package manager(npm) installed.
Step 3
Create a folder “REST_API”
cd REST_API
npm init
When you are prompted for the below, hit enter
package name: (rest-api) version: (1.0.0) description: entry point: (index.js) test command: git repository: keywords: author: license: (ISC)
This generates a package.json file inside your REST_API folder.
Run the commands
npm install -g express-generator
npm install express –save
Express is a really cool Node framework that’s designed to help JavaScript developers create servers really quickly. NodeJS may be server side.
Step 4
Open the folder REST_API in VS Code. If you don’t have it, install it from here.
Then create the app.js file or whatever you prefer naming it (default is index.js) and add in the following code.
var express = require("express"); var app = express(); app.listen(3000, () => { console.log("Server running on port 3000"); });
We just created a Waiter in the restaurant.
Now let us tell the waiter what to get from the kitchen by adding the following code.
app.get(“/url”, (req, res, next) => { res.json([“Tony”,”Lisa”,”Michael”,”Ginger”,”Food”]); });
.
We have now specified what the Waiter needs to fetch.
Let us sit at the table and order → Run this simple application
Open the terminal and run the command
npm app.js
You will see the message – “Server running on port 3000”
On any browser, go to the address, http://localhost:3000/url
You should see the below.
What we did here is
- We created a simple waiter for our restaurant
- We told the waiter what to get
Since this is a simple app, the return result is also placed in the same app.js but for an actual application, the results would be fetched out of the database.
I hope this explanation for creating a simple REST API was easy to understand.
The objective is to show you what an actual REST API is.
Now that we know what REST API is, let us understand what a GraphQL API is all about.
Assume we need 10 items to be fetched from the kitchen.
With REST API , we will need to tell the waiter multiple times to get different items.
We will be making multiple calls to the application on the other end – which is mostly a database.
We solve this by creating a GraphQL API. A GraphQL API will be able to fetch all what we need in a single call.
GraphQL follows WYAIWYG pattern – What You Ask Is What You Get.
To understand this, let us first build a simple GraphQL API. This is not a comprehensive tutorial on GraphQL APIs and hence we won’t be getting into its specifics. All we care about is understanding how to test API. For this, we need to visualize this animal called GraphQL.
A Simple GraphQL Application
Users — Movies — Reviews
There are multiple users.
A user can watch multiple movies and write multiple reviews.
We will just create a simple API to fetch the list of users. Instead of creating a database, we will just add all details to a javascript file called fakeDatabase.js and use this as our database.
We will be using a simple GraphQL framework called graphql-yoga.
Step 1
Create a folder called GraphQLProject
cd GraphQLProject
npm init
npm install graphql-yoga
Step 2
Create a sampleData.js file inside the src folder with the following contents. If there is no src folder, create it.
const users = [ { id: '1001', name: 'User 1', email: 'user1@example.com', }, { id: '1002', name: 'User 2', email: 'user2@example.com', }, { id: '1003', name: 'User 3', email: 'user3@example.com', } ] const movies = [ { id: '2001', title: 'Goldeneye', reviews: '3001', }, { id: '2002', title: 'Wreck-It Ralph', reviews: '3002', }, { id: '2003', title: 'Lego Movie', reviews: '3002', } ] const reviews = [ { id: '3001', movie: '2001', reviewText: 'Goldeneye review...', rating: 4, user: '1002', }, { id: '3002', movie: '2002', reviewText: 'Wreck-It Ralph review...', rating: 5, user: '1001', }, { id: '3003', movie: '2001', reviewText: 'Goldeneye review 2...', rating: 2, user: '1002', }, { id: '3004', movie: '2003', reviewText: 'Lego Movie review...', rating: 5, user: '1002', } ] module.exports = { users, movies, reviews, }
This is our sample – fake database.
Step 3
Create an index.js file within the src folder and add the following content to it.
const { GraphQLServer } = require('graphql-yoga') const { users, movies, reviews } = require('./sampleData') // Scalar types: String, Int, Float, Boolean, ID const typeDefs = ` type Query { users: [User]! } type User { id: ID! name: String! email: String! } ` const resolvers = { Query: { users(parent, args, context, info) { return context.users } } } const server = new GraphQLServer({ typeDefs, resolvers, context: { users, movies, reviews } }) server.start(() => console.log('Server is running on localhost:4000'))
Step 4
Now open the terminal and run the command
node src/index.js
Our GraphQL server is running at port 4000 this time.
Open localhost:4000 in your browser
This is a user interface you can use to send queries to the GraphQL server.
We will write a simple Query to fetch all users.
This will fetch us the results as below
Congratulations.
We just created a GraphQL API that fetches a list of users from the database, in this case a fake database.
Now let us understand how to test this.
Testing a GraphQL API
Options: RestAssured, RestSharp, Karate, TestGraphQL
Steps to test on Rest Assured
Rest Assured for GraphQL code sample
We will be testing the GraphQL API at this link
https://api.spacex.land/graphql/
Play around with the SpaceX GraphQL API at the above link.
The code sample can be obtained at https://github.com/maheshtestsigma/RestAssuredForGraphQL
Steps to run this code
- Download folder or Git clone https://github.com/maheshtestsigma/RestAssuredForGraphQL.git
- Open this code on Intellij IDEA or Eclipse
- Allow Eclipse or Intellij to import the dependencies
- Open the BasicGraphQLTest.java
- Run it
The results are as follows:
Steps to test on Rest Sharp
1.Convert the GraphQL query into Stringified JSON format – click here to do it online
2.Secondly, pass the converted String as Body parameters to the Request
3.Validate the response
[SetUp]
public void Setup()
{
_restClient = new RestClient("http://localhost:4000");
}
[Test]
public void TestGraphQL()
{
//Arrange
_restRequest = new RestRequest("/graphql" , Method.POST);
_restRequest.AddHeader("Content-Type", "application/json");
_restRequest.AddParameter("application/json",
"{\"query\":"query{\n users{\n name\n }\n}",
ParameterType.RequestBody);
//Act
_restResponse = _restClient.Execute(_restRequest);
//Assert
//do validation
Console.WriteLine("Printing results for fun : "+_restResponse.Content);
}
Steps to test on TestGraphQL
Add the below maven dependency to your Java project and you are done.
com.vimalselvam test-graphql-java 1.0.0
A sample test can be found here https://github.com/vimalrajselvam/test-graphql-java/blob/master/src/test/java/com/vimalselvam/graphql/TestClass.java
Read more here – Graphql vs Rest.
What is GraphQL Testing?
GraphQL testing validates GraphQL APIs to ensure they work correctly, efficiently, and securely. It involves evaluating various aspects of a GraphQL API, such as its schema, resolvers, queries, mutations, and subscriptions, to verify that they meet the intended requirements and behave as expected.
Follow some tips for effective GraphQL testing:
- Start testing early in development.
- Use a mixture of testing methods, including manual and automated testing.
- Use testing frameworks and tools designed explicitly for GraphQL.
- Collaborate with other developers and stakeholders to ensure that all aspects of the API are tested.
- Continuously test the API as it evolves.
By following these tips, you can ensure that your GraphQL APIs are thoroughly tested and reliable.
What Needs to be Tested in the GraphQL Test?
Here are some critical aspects of GraphQL testing:
- Schema validation: Ensuring the GraphQL schema correctly defines and matches the expected structure. This includes checking the types, fields, and their relationships.
- Query testing: Verifying that GraphQL queries return the expected data and follow the defined schema. This involves testing queries for correctness and completeness.
- Mutation testing: Testing GraphQL mutations to ensure they correctly modify data and handle errors appropriately. This includes testing both successful and error scenarios.
- Performance testing: Assessing the performance of GraphQL queries and mutations to ensure they meet performance requirements. This may involve load testing to determine how the API behaves under various traffic levels.
- Security testing: Identifying and addressing potential security vulnerabilities such as authorization bypass, data leaks, or denial-of-service (DoS) attacks.
- Error handling: Ensuring that error responses from the GraphQL API are informative and follow a consistent format for better client-side error handling.
- Regression testing: Continuously testing GraphQL APIs as they evolve to catch any regressions or unintended behavior introduced by code changes.
- Integration testing: Verifying that the GraphQL API interacts correctly with its underlying data sources, databases, and external services.
- Documentation testing: Ensuring that the API documentation, including descriptions of types, fields, and operations, accurately reflects the API’s behavior.
- End-to-end testing: Testing the complete GraphQL API from client to server to ensure it functions as expected in a real-world scenario.
GraphQL testing can be performed manually or automated using frameworks and tools designed specifically for GraphQL. Effective GraphQL testing is essential to the development process, helping teams catch issues early, deliver high-quality APIs, and provide a reliable experience to clients and end-users.
The Importance of Testing GraphQL APIs
GraphQL API testing requires test cases that can query and mutate nested data.
For example, consider the nested structure of components in a Facebook feed:
This nesting of data is similar to the complexity of shadow DOM. In contrast to REST APIs, which require multiple query parameters and endpoints to communicate, GraphQL’s ability to retrieve interconnected data is particularly beneficial for testing nested data.
Here are some examples of test cases for GraphQL API testing of nested data:
- Query for all posts in a user’s timeline, including the post’s likes, comments, and shares.
- Query for all comments on a particular post, including the commenter’s name and profile picture.
- Mutate a post to add a new comment.
- Mutate a like to remove a like from a post.
By testing nested data in GraphQL APIs, developers can ensure their APIs can handle complex data structures correctly.
Components to Test in GraphQL
Here are the components:
Schema: At the core of GraphQL APIs, the schema defines the available data types, fields, and operations. Schema testing ensures the schema adheres to GraphQL specifications and functions correctly with all queries and mutations.
Query: Query testing involves validating that a specific query and associated parameters generate the expected response. Automated testing methods, such as libraries like “request” and “supertest,” can be employed for query testing.
Mutations: Testing mutations is crucial, as they involve verifying the ability to access and modify database data. Mutations alter data on the server side and return a response.
Resolvers: Resolvers are functions responsible for mapping GraphQL queries to data sources, fetching the data, and returning it in the correct format. Testing resolvers early in development is essential to prevent costly errors later.
If you’re transitioning from REST to GraphQL, here’s how GraphQL testing differs:
Query-Based Testing: GraphQL testing involves interacting with resources by sending GraphQL queries instead of requesting specific endpoints.
Data Fetching and Response Handling: Since clients can specify their data requirements, testing focuses on the response data and the structure of a given query.
Nested Query Complexity: Multi-level nested queries can consume excessive resources, leading to over-fetching or under-fetching data. So, this aspect needs to be verified while testing.
Error Handling: GraphQL introduces a separate field for errors in the response body instead of relying solely on HTTP status codes and error messages for error handling.
Types of GraphQL API testing
There are several key types of testing for GraphQL API testing:
- Functional Testing: It focuses on specific functions within the API to ensure that the actual output aligns with expected outcomes. For example, when testing an API’s login feature, a test case might confirm that users can successfully log in with valid credentials while being denied access with invalid ones.
- Security Testing: To safeguard GraphQL APIs from security threats like injection attacks, information leakage, and inconsistent authorization, it’s crucial to conduct security testing. This includes verifying encryption methods and evaluating the design of API access control.
- Load Testing: Load testing assesses how well the API performs under a high volume of requests. It’s essential to ensure the API can handle expected user traffic in various scenarios, such as peak usage, unexpected traffic spikes, and prolonged usage.
- Validation Testing: Validation testing checks the API’s usability, transactional behavior, and operational efficiency. Typically conducted during the final stages of development, this type of testing assures the overall functionality of the API.
- Unit Testing: This level involves testing individual units or components of the API in isolation. In the context of GraphQL, unit tests are used to evaluate individual resolvers and fields within the schema.
- Integration Testing: Integration testing validates the API and the front-end user interface interaction. It ensures that data is correctly consumed and displayed by the UI.
- End-to-end Testing: End-to-end testing replicates a sequence of requests & responses between the client and server. In the case of a GraphQL API, this type of testing covers the entire lifecycle, encompassing aspects from schemas and resolvers to queries and responses.
By implementing and adhering to these testing strategies, you can thoroughly assess and verify the functionality, security, performance, and overall reliability of your GraphQL API.
Testing GraphQL Best Practices
Here are some best practices for GraphQL API testing:
- Isolate tests and maintain independence: Each test case should be independent of the others so that you can easily identify the cause of test failures and run tests in parallel.
- Use mocking for efficiency: Mock database interactions, third-party services, and external dependencies to speed up test execution and make tests more predictable.
- Test query scenarios: GraphQL clients can request specific data, so test different query scenarios to verify that your API responds correctly to various client requests, including complex nested queries and multiple endpoints in a single request.
- Test error handling: GraphQL APIs often return structured error responses. Include test cases for invalid queries, unauthorized requests, and server-side errors to ensure the API returns appropriate error codes and messages.
- Implement CI/CD: Integrate your GraphQL API tests into your CI/CD pipeline to automatically run tests with every code change. This helps catch issues early and ensures that only reliable code is deployed to production.
- Monitor and analyze test coverage: Track test coverage metrics to identify areas of the schema that may be under-tested. This helps ensure that critical parts of your GraphQL schema are thoroughly tested.
- Consider performance testing: Besides functional and security testing, consider performance testing to determine how well your GraphQL API performs under load. Use tools like Apache JMeter or Gatling to simulate high-traffic conditions and identify bottlenecks.
- Document and share test cases: Document your test cases and share them with the development team and stakeholders. Clear documentation helps everyone understand the expected behavior of the API and facilitates collaboration in identifying and resolving issues.
- Version control for test data: If your tests require specific test data, maintain this data in version control. This ensures that the test data is consistent across environments and allows for easy data setup for new team members or testing environments.
- Regularly update tests: As your GraphQL API evolves, update your tests to reflect the changes in the schema and functionality. Keeping tests up to date ensures that they continue to provide accurate feedback on the API’s behavior.
By following these best practices, you can establish a robust testing strategy for your GraphQL API that improves its reliability, security, performance, and maintainability.
GraphQL Testing Tools
Here are some of the best GraphQL testing tools you can use.
Testsigma
Testsigma is a test automation tool that makes GraphQL easier to use. GraphQL enables you to communicate and execute queries using pre-existing data.
Postman
Postman, a popular API development tool, can test GraphQL APIs. It allows you to create and run GraphQL queries and mutations, making it useful for development and testing.
Insomnia
A powerful HTTP client with dedicated GraphQL support, allowing testing and collaboration.
TestCafe GraphQL
An open-source library for writing automated GraphQL tests with TestCafe, offering a comprehensive testing experience.
Automate Your GraphQL Tests with Testsigma
Let’s take a closer look at Testsigma, one of the most popular automation testing tools available.
To test APIs using GraphQL queries, navigate to API Request > Body > GraphQL.
1.After you select GraphQL, you will see two sections: Query and Variables, as shown in the image above. Now, enter your GraphQL Query into the Query field.
NOTE: When selecting GraphQL, the request method is, by default, set to POST.
2.After entering the query, adding variables can enhance query flexibility. You can define these variables in either JSON format or using the Table option in the Variables section.
NOTE: You can optionally use variables in GraphQL.
3. When adding variables in JSON format, enter keys and variables in the form { “code”: “US” }.
4. Alternatively, when you select the Table format for adding variables, you can conveniently select Keys from a dropdown menu and provide corresponding Values. You can include Test Data (Parameter, Environment, Runtime) in the Value section of the table to try out different scenarios with different variable values.
5.Click the Send button to initiate the request once you have set up the queries and variables. This action will result in a JSON Response.
6. Finally, you can Store Variables or Add Verification for validation after generating the JSON Response. The specified test data values will trigger corresponding responses during the execution of test cases.
What is GraphQL Compared to REST?
Let us take an example and compare GraphQL and REST to understand both better.
Imagine you’re at a restaurant. Here’s how ordering food compares to REST and GraphQL:
REST:
- Menu: You get a physical menu with every dish listed.
- Ordering: You tell the waiter what dishes you want from the menu, one by one.
- Delivery: You get each dish separately, maybe with unwanted extras (like fries when you only ordered the burger).
GraphQL:
- Menu: You tell the chef exactly what you want in your dish, down to the spices and toppings.
- Ordering: You write a single order note with all your preferences.
- Delivery: You get a single, custom-made dish with exactly what you requested, no waste.
Key differences:
- REST: Fixed data structure, multiple requests needed for different parts.
- GraphQL: Flexible data structure; single requests can get all desired information.
Benefits of GraphQL:
- Efficient: Gets only what you need, no unnecessary data.
- Flexible: You choose exactly what you want, no over-fetching.
- Precise: No surprises; you get exactly what you ordered.
- Evolving: Adapts to changing needs easily.
💡REST is like a traditional restaurant with a set menu, while GraphQL is like a custom kitchen where you get precisely what you crave.
Frequently Asked Questions
How to test GraphQL in the browser?
To test GraphQL in the browser, you can use a GraphQL IDE such as GraphiQL. GraphiQL provides a graphical interface for writing, validating, and testing GraphQL queries and mutations.
Does JMeter support GraphQL?
JMeter’s GraphQL HTTP Request sampler is a more comfortable and easier way to test GraphQL APIs, but you can still use the HTTP Sampler if you prefer.
What is the best tool for testing GraphQL?
The choice of the best tool for testing GraphQL depends on your specific testing requirements, workflow, and preferences. Several tools, like Postman, GraphiQL, GraphQL Playground, Katalon, Testsigma, etc., are available for testing GraphQL APIs, each with its strengths and use cases.